Make a URL Shortener that is Simple Utilizing Python (r) (r)
URL Shortener
Enter an https:/ URL: Enter Created URL"/form>" Body>/html>
The code above creates an online form that has two labels and two fields for input as and one button.
The first input field, dubbed URL
, is where you write your long URL, and the other field will generate the shorter URL.
The URL
input field contains these characteristics:
name
In order to identify this element ( e.g. URL)placeholder
: To show a URL for an example- pattern: To specify the pattern of an URL that is https ://. *
is necessary
: To give an input URL prior to transmittingvalue
Take a look at the URL previously utilized
In the second field, there is a value attribute. comes with an value
attribute that is used to define the new_url attribute.
. New_url an uncomplicated URL.
is an uncomplicated URL generated by the shorteners pyshorteners library, which is located in the main.py file (shown in the following section).
The entry form is identified by this picture:
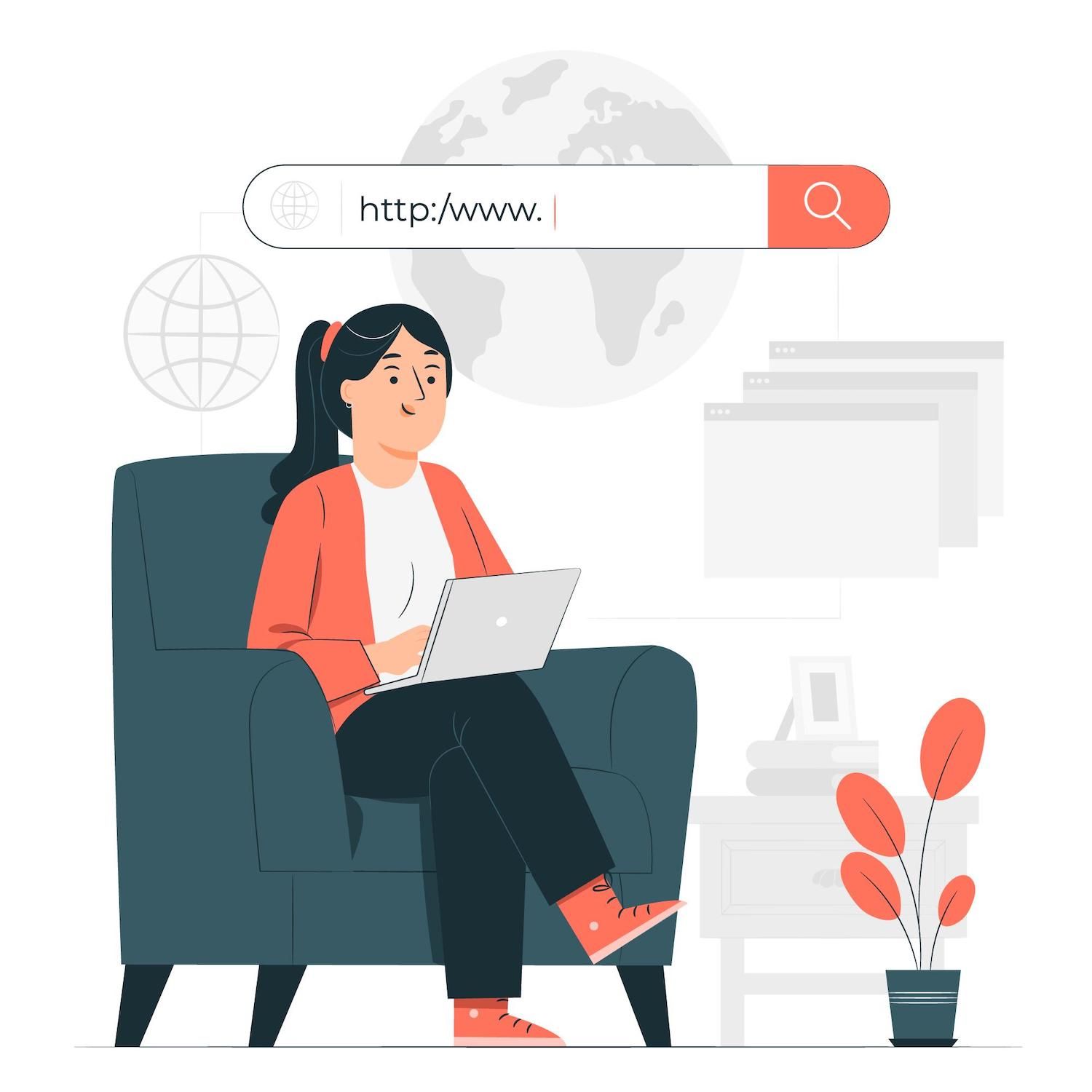
URL Shortening Code Using pyshorteners
When you've completed the form, you'll be able to make it more functional using Python and shorteners in Python.
The code will convert the long URL to a shorter one and run the web application. Open your main.py file you made earlier and add the code below, and save it as:
from flask import Flask, render_template, request import pyshorteners app = Flask(__name__) @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] short_url = pyshorteners.Shortener().tinyurl.short(url_received) return render_template("form.html", new_url=short_url, old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
The code above imports the shorteners the pyshorteners library and the following modules that are part of the Flask framework and will be required to shorten URLs:
Flask
the Flask framework, itself, was previously introduced.render_template
The HTML0 render template is a rendering package that generates HTML files. HTML documents are generated from directorytemplates
.request
A class in the Flask framework that holds the entire data the user sends via the front end of the application to the backend such as the HTTP request.
It then creates an application called home()
that takes URLs submitted on the form and creates an abbreviated URL. Its app.route()
decorator can be used to connect the function to a specific URL route to run this application. The POST/GET methods deal with the request.
Within"home()", the "home()
function, you will find an between
conditional statement.
For the if
statement, if request.method=="POST"
, a variable called url_received
is set to request.form["url"]
, which is the URL submitted in the form. It is so because URL
is the name of the input field specified in the HTML form which was designed earlier.
Then, a variable called short_url
is set to pyshorteners.Shortener().tinyurl.short(url_received)
.
Two methods use the pyshorteners library: .Shortener()
and .short()
. The .Shortener()
function creates an pyshorteners class instance and the .short()
function is used to input the URL for an input and shortens the URL.
The brief()
function, tinyurl.short()
is one of the shorteners that are part of the pyshorteners libraries many APIs. osdb.short()
is an additional API that can be utilized to accomplish the same purpose.
This render_template()
function is utilized to produce the HTML template file form.html and send URLs back to the form using arguments. This function uses arguments. "new_url"
argument is changed as short_url
as well as the argument for old_url
is set to the URL that is receiving
. The is
declaration's scope is now over.
If you use the else
statement, in the event that the request method differs from POST, it will be displayed using the form.html HTML template will be shown.
A demonstration of URL Shortener Web App built with the Python Shorteners Library
To demonstrate the pyshorteners URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Enter a hyperlink you like on the top of the form for web:
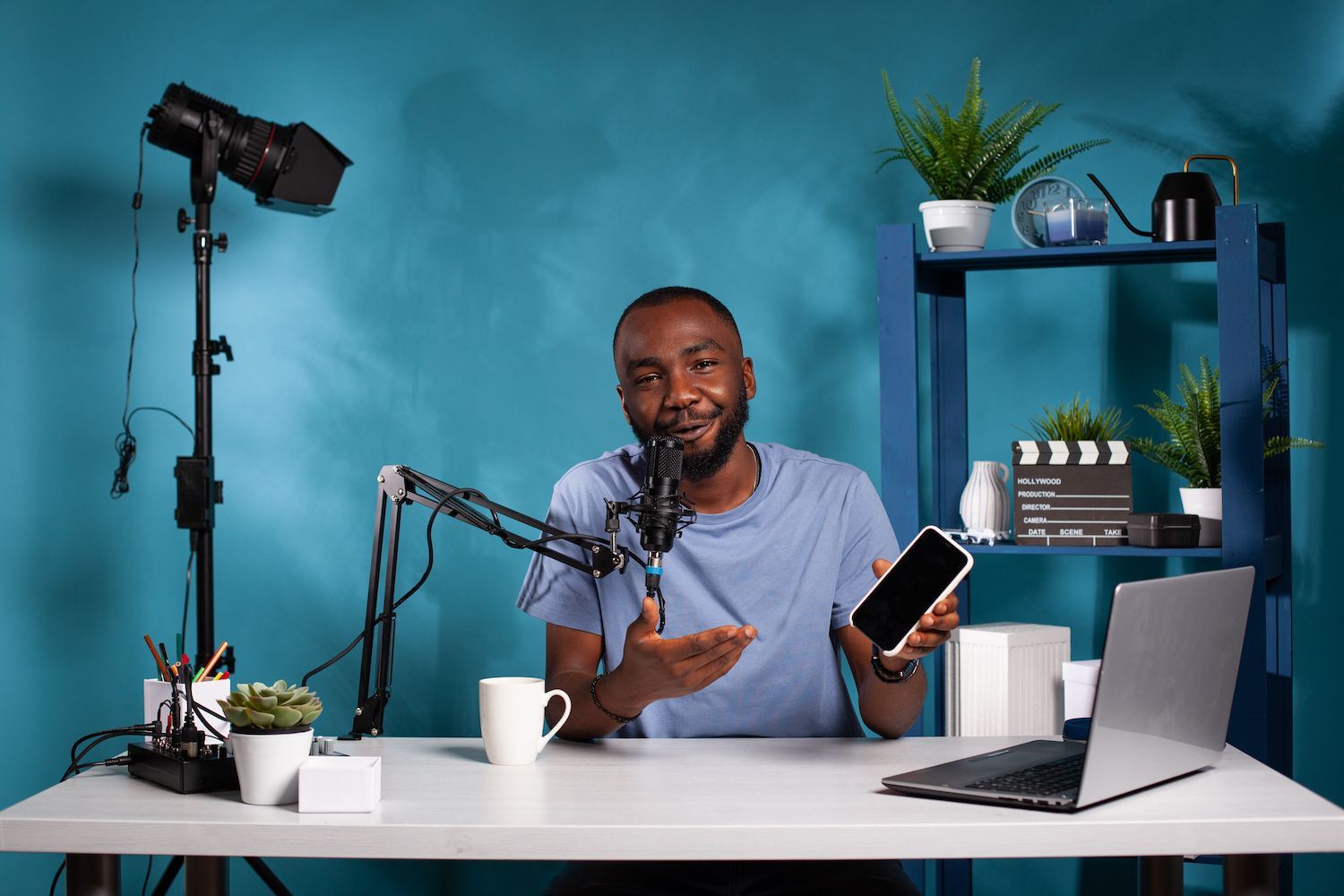
Press the Submit button to generate URL. Click Submit to output the URL in a smaller version, utilizing tinyurl
as the domain name in the field that says created URL
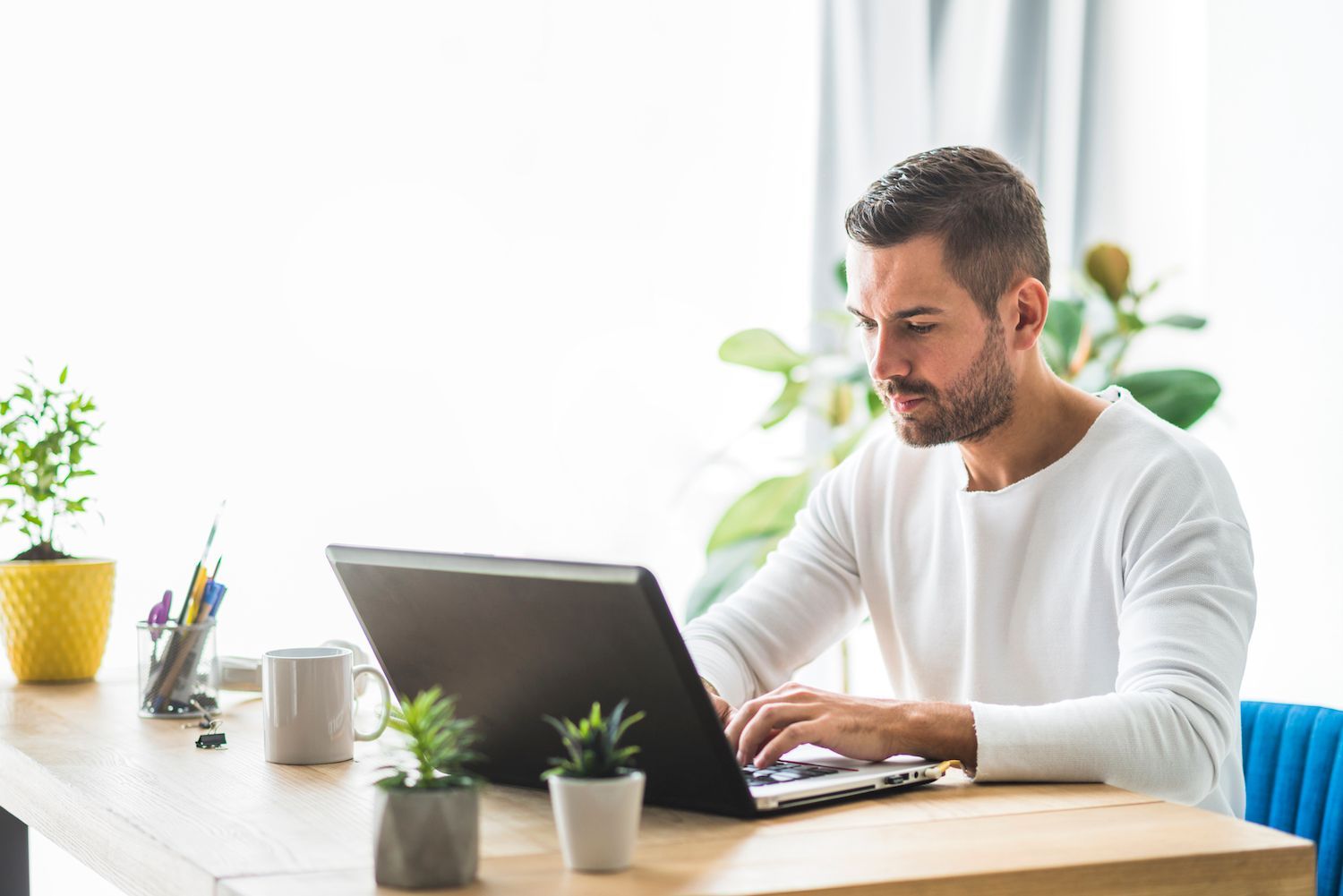
Utilizing Bitly API Module Bitly API Module, you'll be able to build an URL Shortener App for the Web. App
In this section, you'll develop a URL-shortening application utilizing Bitly API. Bitly API. The Bitly API module is another method for shortening URLs and offers comprehensive statistics of clicks, location as well as the type of device being utilized (such as desktop or mobile).
Install Bitly API. Download the Bitly API using the following procedure:
pip for installing Bitly API-py3
Access tokens are required for login to Bitly API. They are accessible access tokens by logging in to Bitly.
When you've completed the signup procedure. After you've completed the sign-up process, login to Bitly to view your dashboard
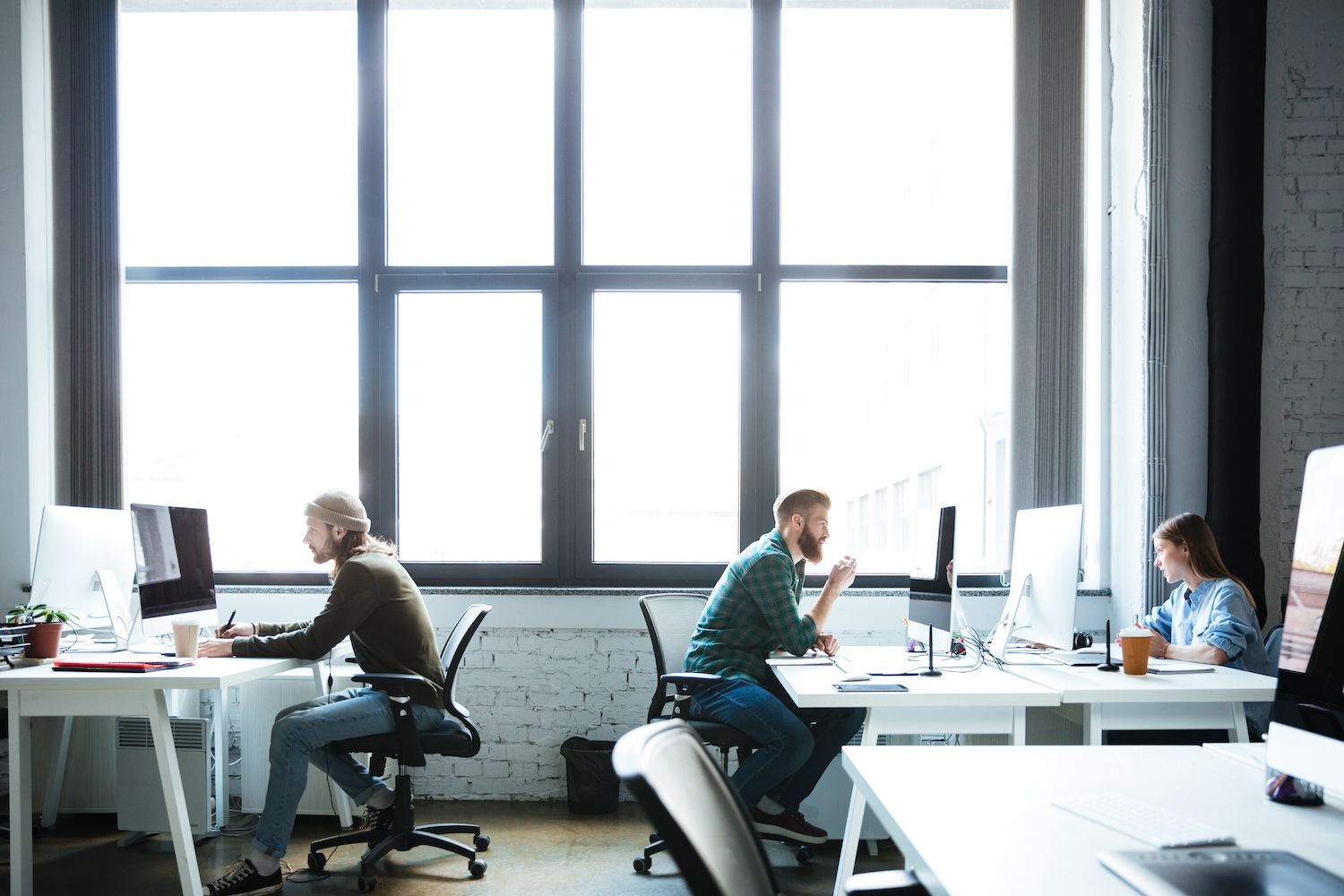
Select Settings on the left sidebar, then navigate through the API section that is in Developer settings.
Generate an access token by entering your password in the area just above button Generator token button, which can be seen in the image below. save the token for use in the code for your application:
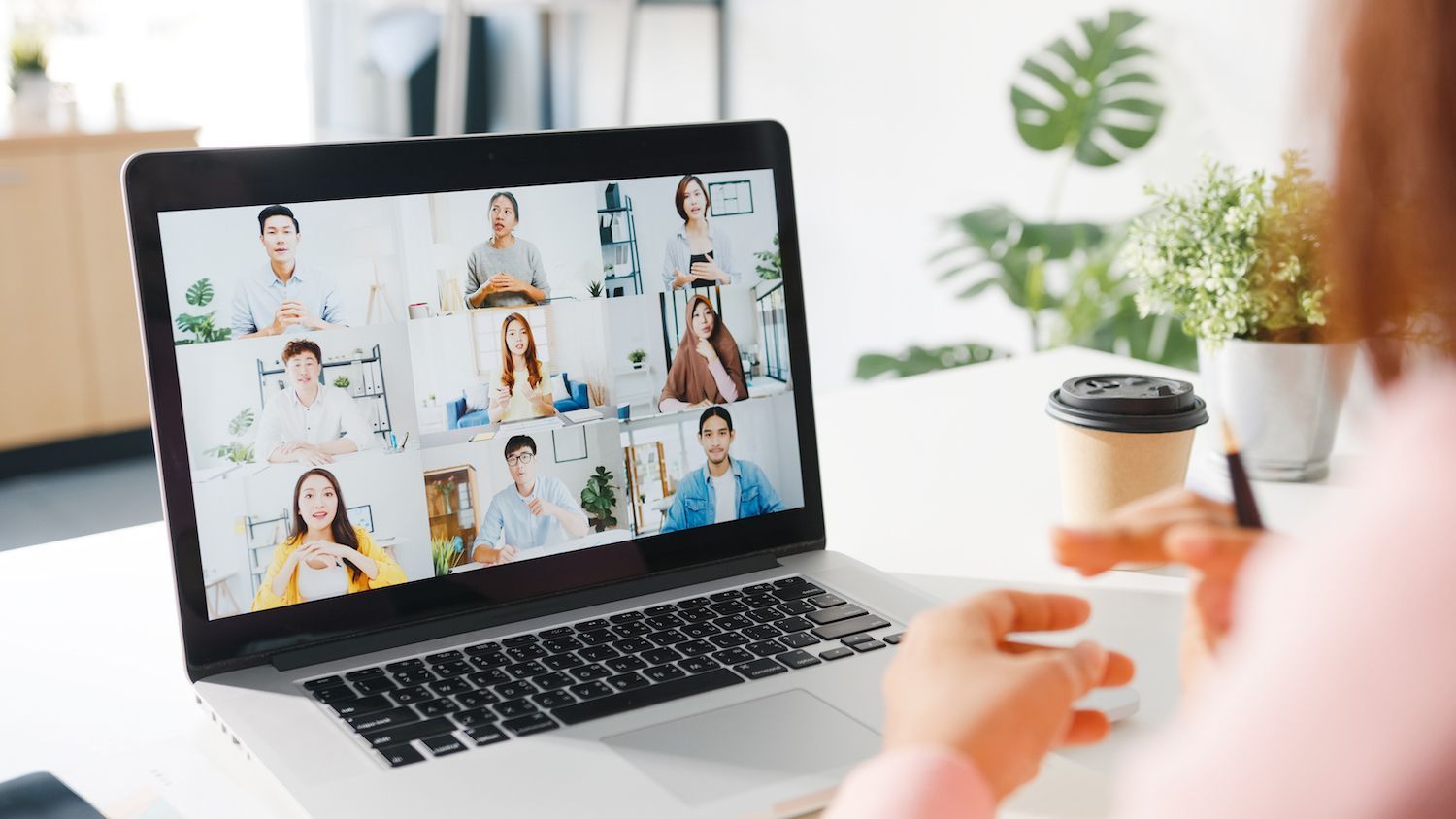
URL Shortening Code Using the Bitly API
When you've received your Bitly token via Bitly Then you can program your web application to reduce the length of the URL by via Bitly API. Bitly API.
The same template you created to create your shortereners section, however with a few changes to the main.py file:
from flask import Flask, render_template, request import bitly_api app = Flask(__name__) bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxxxx" @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] bitly = bitly_api.Connection(access_token=bitly_access_token) short_url = bitly.shorten(url_received) return render_template("form.html", new_url=short_url.get('url'), old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
In the example above, bitly_api
is loaded by using import bitly_api
. The access token is then saved in a variable called bity_access_token
, as in bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxx"
.
The "home"()
function is a shortening of the URL. It also contains an "in-case-of-nothing"
conditional expression.
When you are using the If
statement when POST is the preferred method, or the request uses been formatted as POST
The URL entered on the form will be modified to that of the URL_received
variable.
The bitly_api.Connection(access_token=bitly_access_token)
function connects to the Bitly API and passes it the access token you saved earlier as an argument.
In order to reduce the length of URLs, you can use bitly.shorten() function. bitly.shorten()
function. It can be utilized to reduce the URL by supplying your URL_received
value as an argument and storing the result into a variable called short_url
.
The form will be rendered and URLs are then sent back to display in the form, using the render_template()
function. The when
statement is the final step in the form.
In the other
clause for the else assertion, the model will be rendered with render_template() function. render_template()
function.
A demonstration of URL Shortener Web Application built with the Bitly API
To demonstrate the Bitly API URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Input a link that you like in the first box of the web page form:
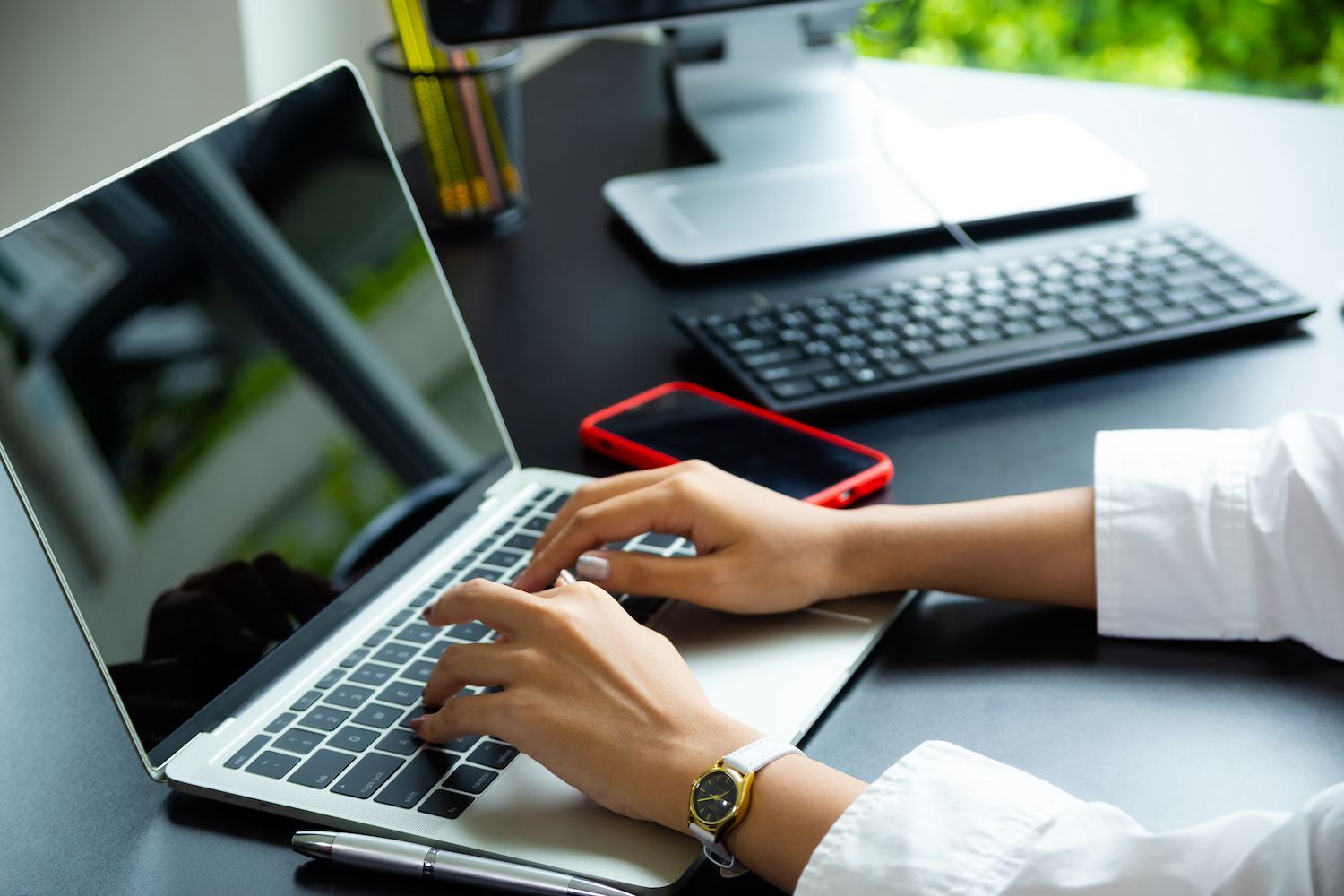
Press "Submit" to generate a short URL that uses bit.ly
as the domain. The second part that is the web app:
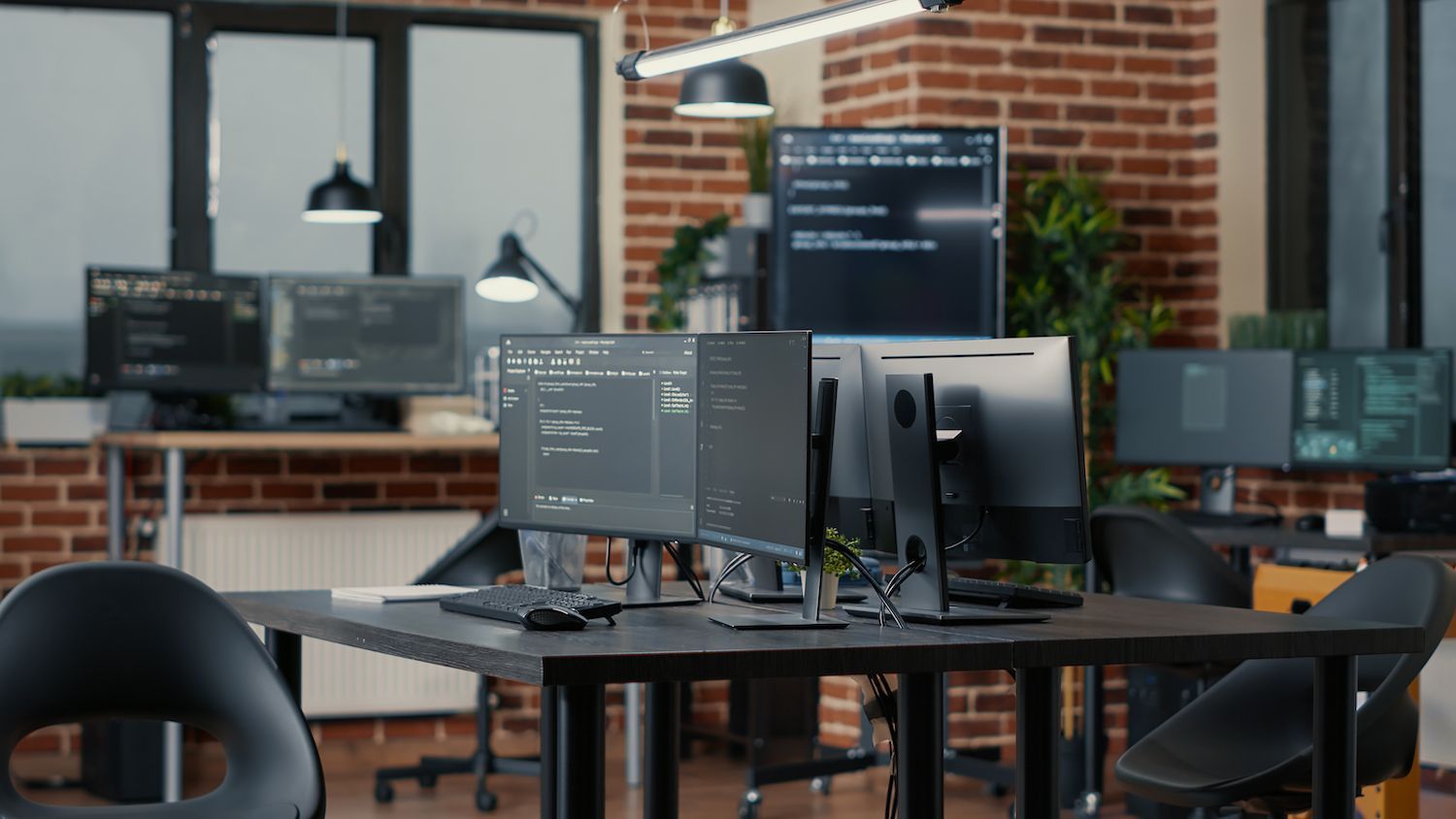
Utilizing the Bitly API to use the Bitly API to shorten URLs in your Python program is as simple as that.
Summary
URL shorteners offer you short URLs users can use easily and are more attractive and take up less space. In this article, you'll learn about URL shorteners, and the benefits they bring and methods to build an URL shortener-related website application using Python by using Pyshorteners and using the Bitly API. It is the Python shorteners library provides shorter URLs. The Bitly API provides detailed analytics along with shorter URLs.
Adarsh Chimnani
Adarsh is a web developer (MERN stack) He is passionate about game-level design (Unity3D), and an avid fan of anime. He is awed by learning out of curiosity, implementing the knowledge he's acquired on the job and then sharing his information with others.
This post was first seen on here