Laravel API Create and test an API to Laravel -(r) (r)
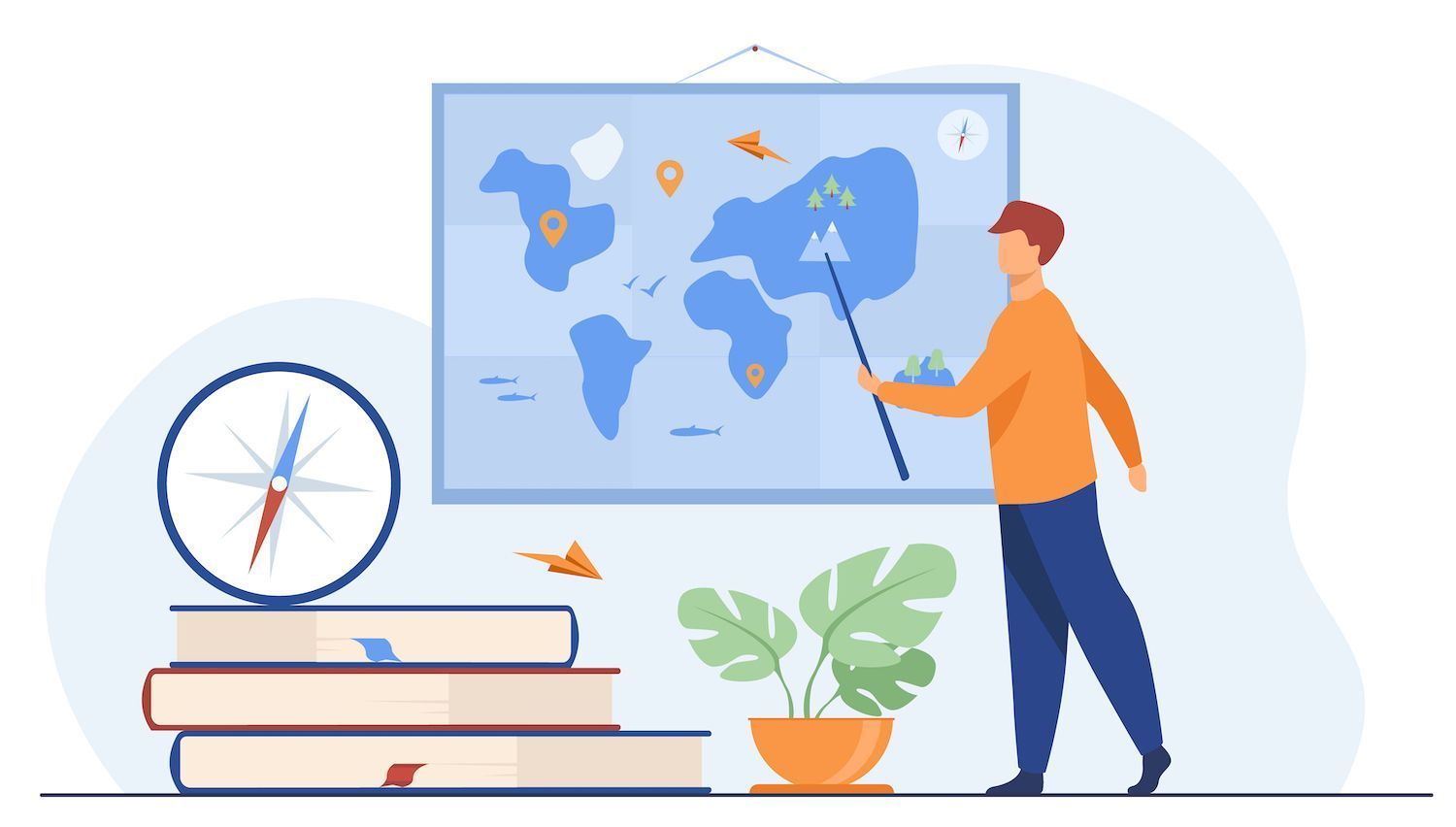
Please forward the news to
Laravel Eloquent can be a straightforward way to interact to your database. It's an object-relational map (ORM) which simplifies the complexity of databases through creating a framework which allows you to interact with tables.
In this way, Laravel Eloquent has excellent tools for creating and testing APIs. These tools can assist you to create APIs. In this article, you'll learn how simple to build and test APIs in Laravel.
The conditions
In order to start Here's the things you'll require:
API Basics
Start by creating an entirely new Laravel project with the composer
:
composer create-project laravel/laravel laravel-api-create-test
To begin the server run this command. This starts the server at port 8000:
cd laravel-api-create-test php artisan serve
It is highly recommended that you check out the following screen:
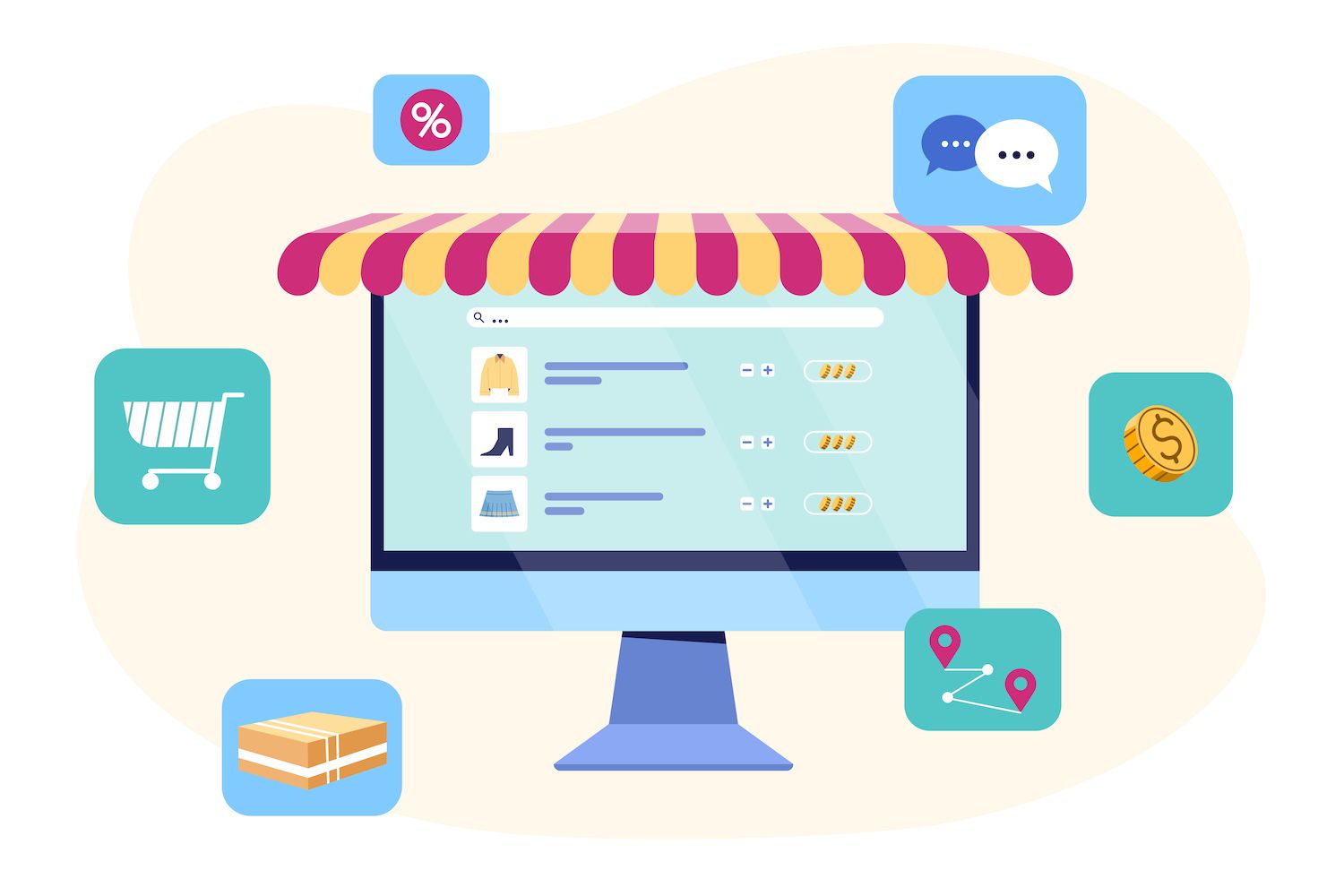
Make models that have an "-m
flag to enable the migration by with the code that is in the following:
php artisan make:model Product -m
Then, upgrade your file in order to include the required field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title'); $table->longText('description');
The next step is to make these fields fully filled. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
How do you make the Controller?
Make a controller file that will control the product with the following command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Create the logic for creating and retrieving objects. Within the index method index
method, you can add this code to look for every item.
Products = $products + All :(); Return the result ()->json(["status" is"false" as well "products" exceeds"$products");
Then, you need to include a StoreProductRequest
class that will store the latest items within your database. The following class is to be put on top of the same file.
public function store(StoreProductRequest $request) $product = Product::create($request->all()); return response()->json([ 'status' => true, 'message' => "Product Created successfully! ", 'product' => $product ], 200);
The following step is to initiate the process of creating the request. You can do by executing this command:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. It's a test that doesn't have validation.
What Do You Need To Create For A Route
The final step before trying using an API is to create the new route. In order to do this, include this code into your routes/api.php file. Include in the file the usage
declaration at the middle of the file, and the Route
assertion within the body
use App\Http\Controllers\Api\ProductController; Route::apiResource('products', ProductController::class);
Before you start testing the API, ensure that you have a products table exists in your database. If not then create the table using the help of a control panel such as XAMPP. Also, you can execute the following commands to transfer the database
php artisan migrate
What is the best way to test an API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
Make a brand new product with Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. Since this is a request to POST an
application for the development of a new product, it is necessary to provide an JSON object that contains the name and description.
"title":"Apple", "description":"Best Apples of the world"
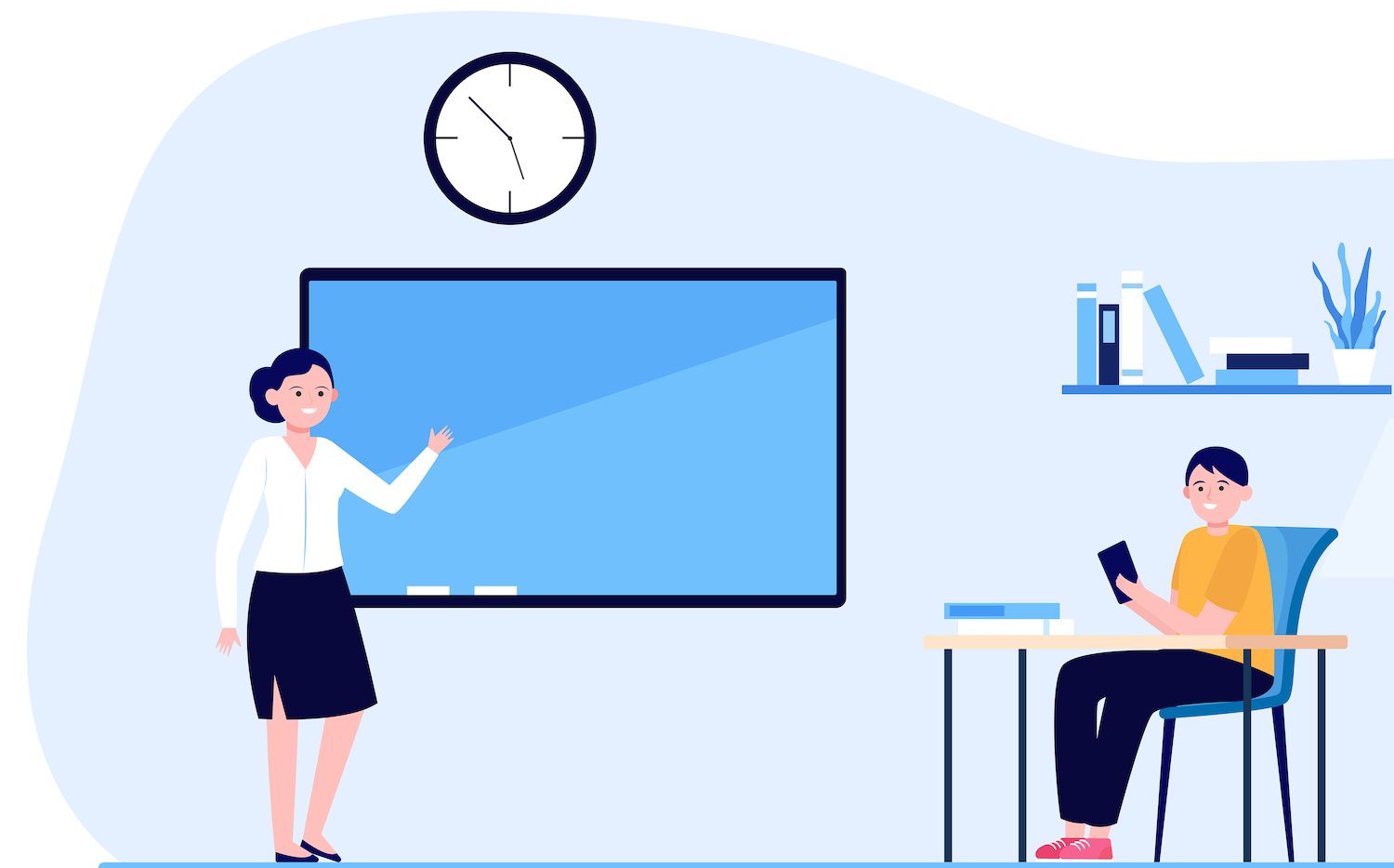
When you've clicked"Send" when you have clicked the "Send" button You will receive this details:
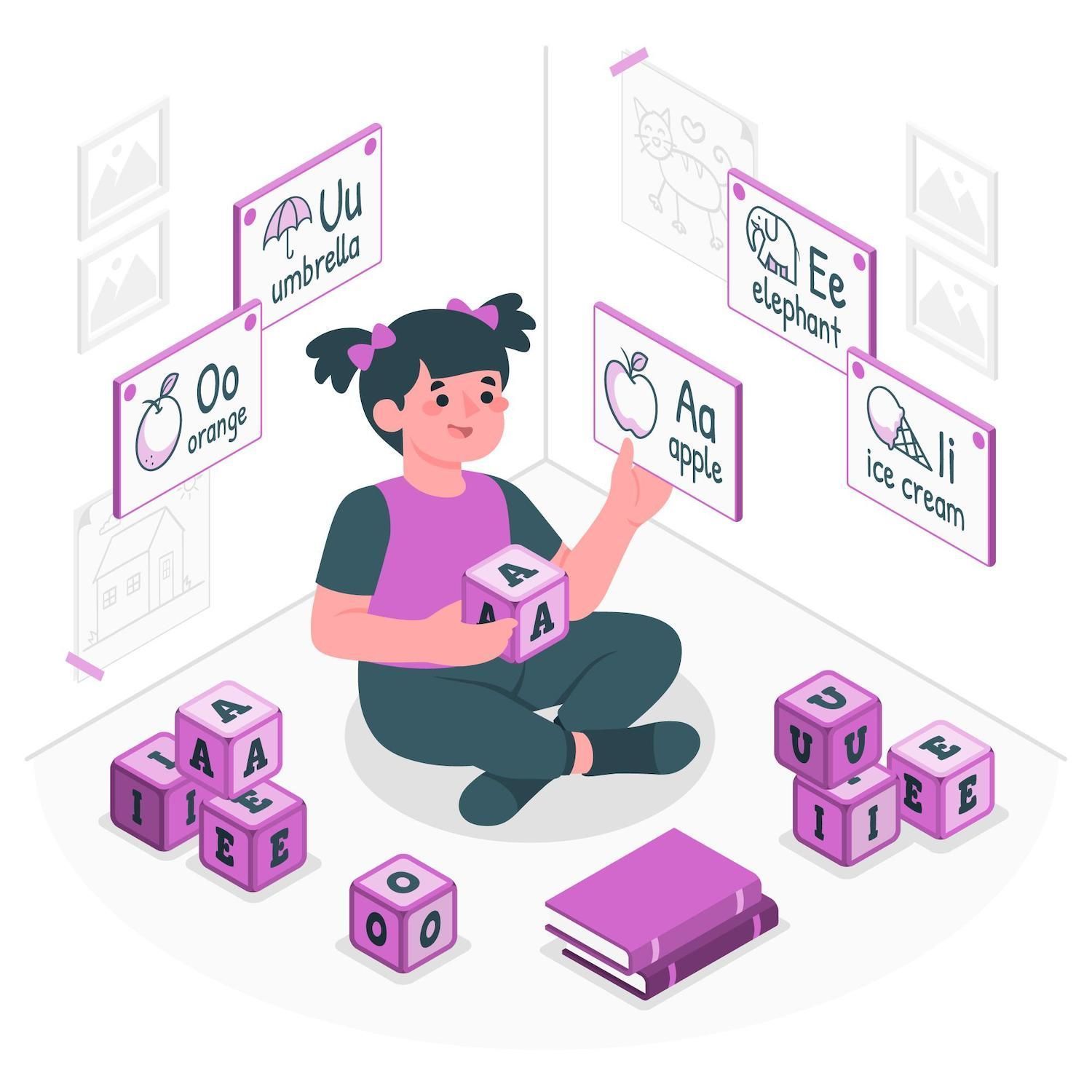
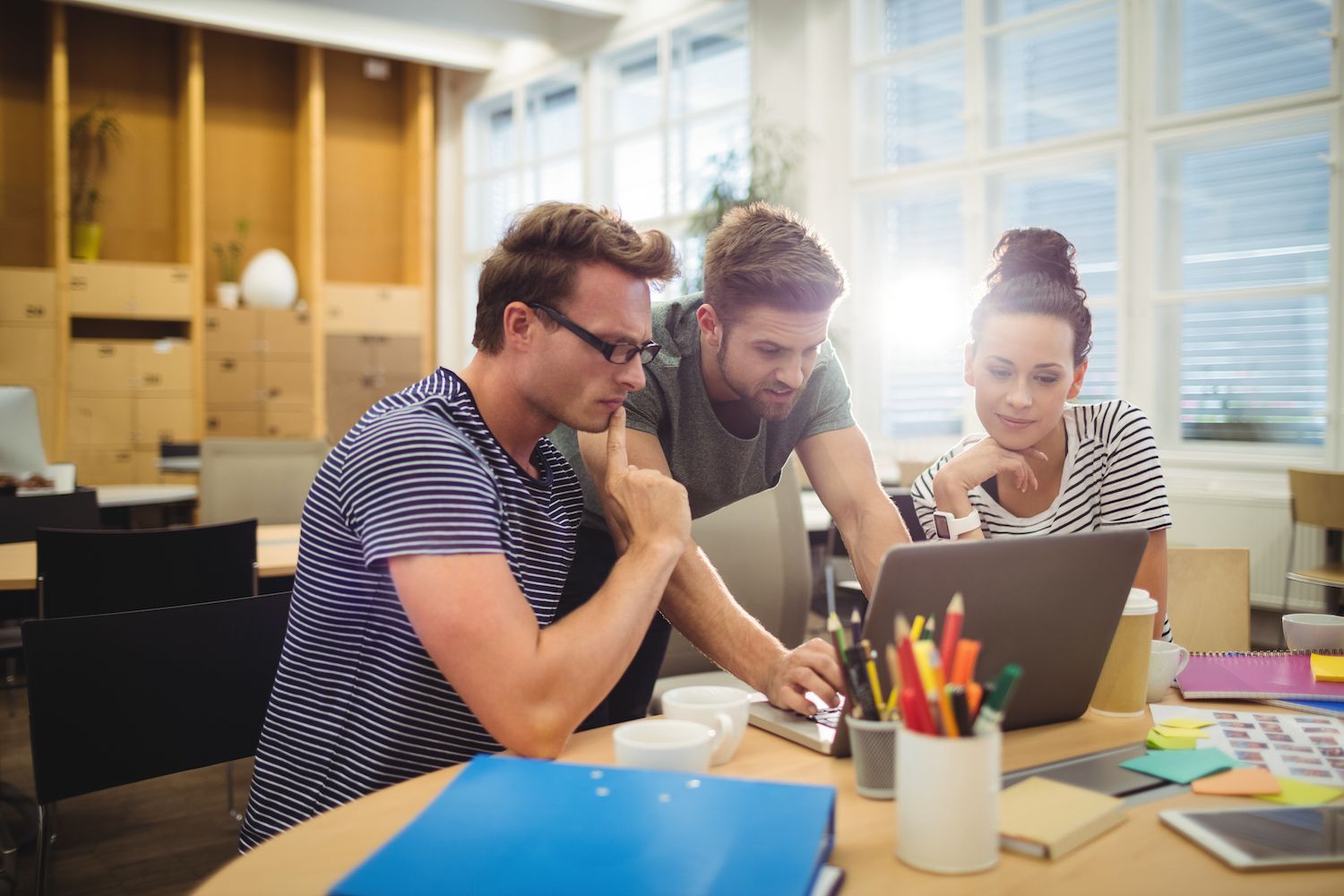
Which is the most efficient method of authenticating APIs using Sanctum?
Security of your API requires authentication. your API. Laravel facilitates it with the feature that is built into the Sanctum token, which could be utilized as an intermediaryware. It protects APIs with tokens created when users log into their accounts using the correct username and password. Make sure that you are aware that the user won't be capable of accessing an API secured by token.
The first step for adding authentication is to add the Sanctum package using the following code:
composer require laravel/sanctum
Make available the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
After that, add Sanctum's token into middleware. Within the app/Http/Kernel.php file, you should use the following class, and substitute middlewareGroups
using the below code to access the protected middleware group' API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [ 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, // \Illuminate\Session\Middleware\AuthenticateSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, \Illuminate\Routing\Middleware\SubstituteBindings::class, ], 'api' => [ EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
The next step is to create the UserController
and then add the code to generate the authentication token.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
Before you try to authenticate it is necessary to establish an account for an individual user, using seeders. This command will create an users table document.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Begin the Seeder now with this guideline:
php artisan db:seed --class=UsersTableSeeder
The final step to complete the authentication process is to implement the middleware designed to secure the routing. Examine the routes/api.php file, and then add the route of your product to it. routes/api.php file and incorporate the product's route into the middleware.
use App\Http\Controllers\UserController; Route::group(['middleware' => 'auth:sanctum'], function () Route::apiResource('products', ProductController::class); ); Route::post("login",[UserController::class,'index']);
After you've created a new route using the middleware it will show an error from the server internals when you attempt to download the products.
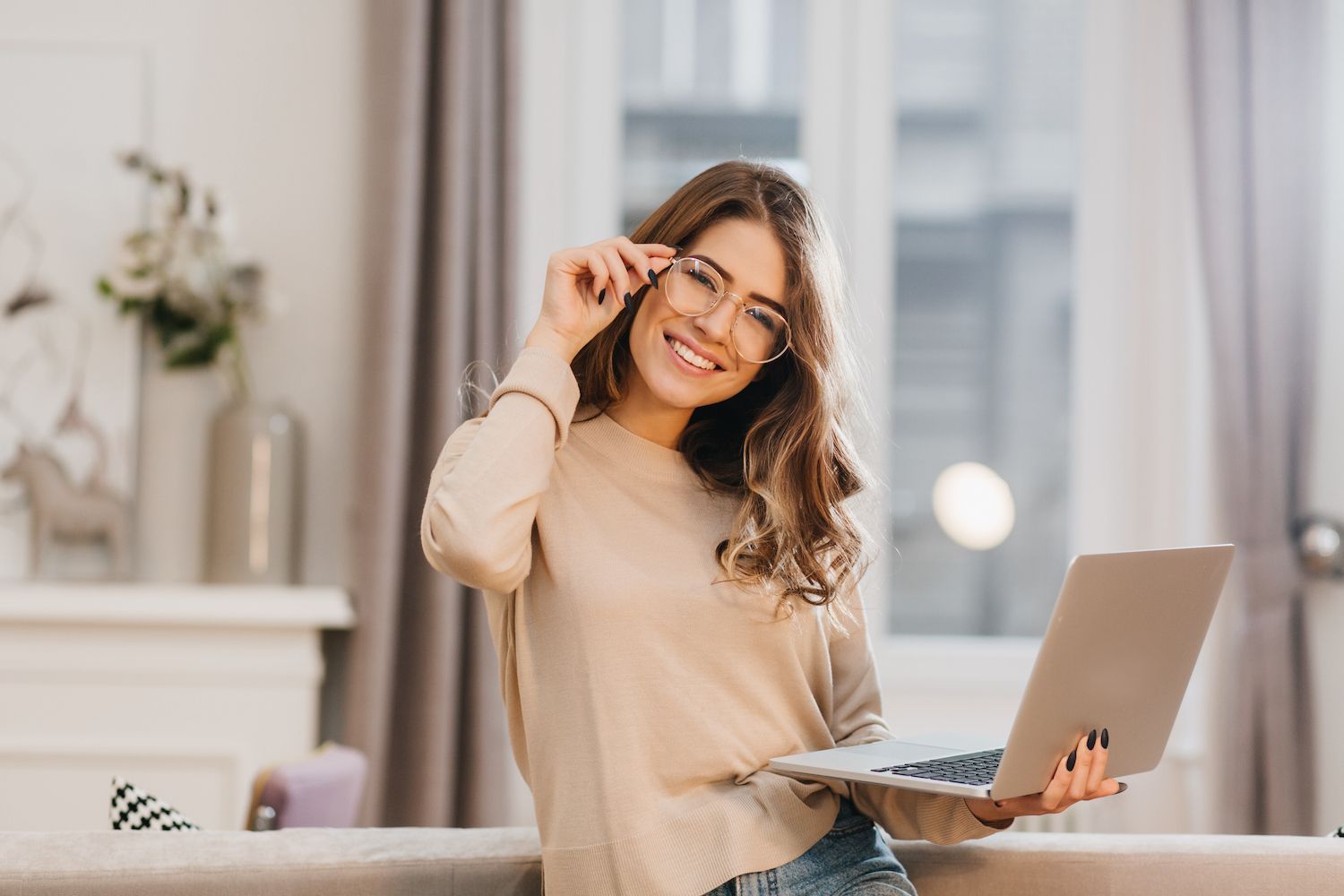
When you've successfully logged in to your account, you'll receive with a unique token to use for your account. If you put it as the HTTP header. It will then verify your identity before working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]", "password":"password"
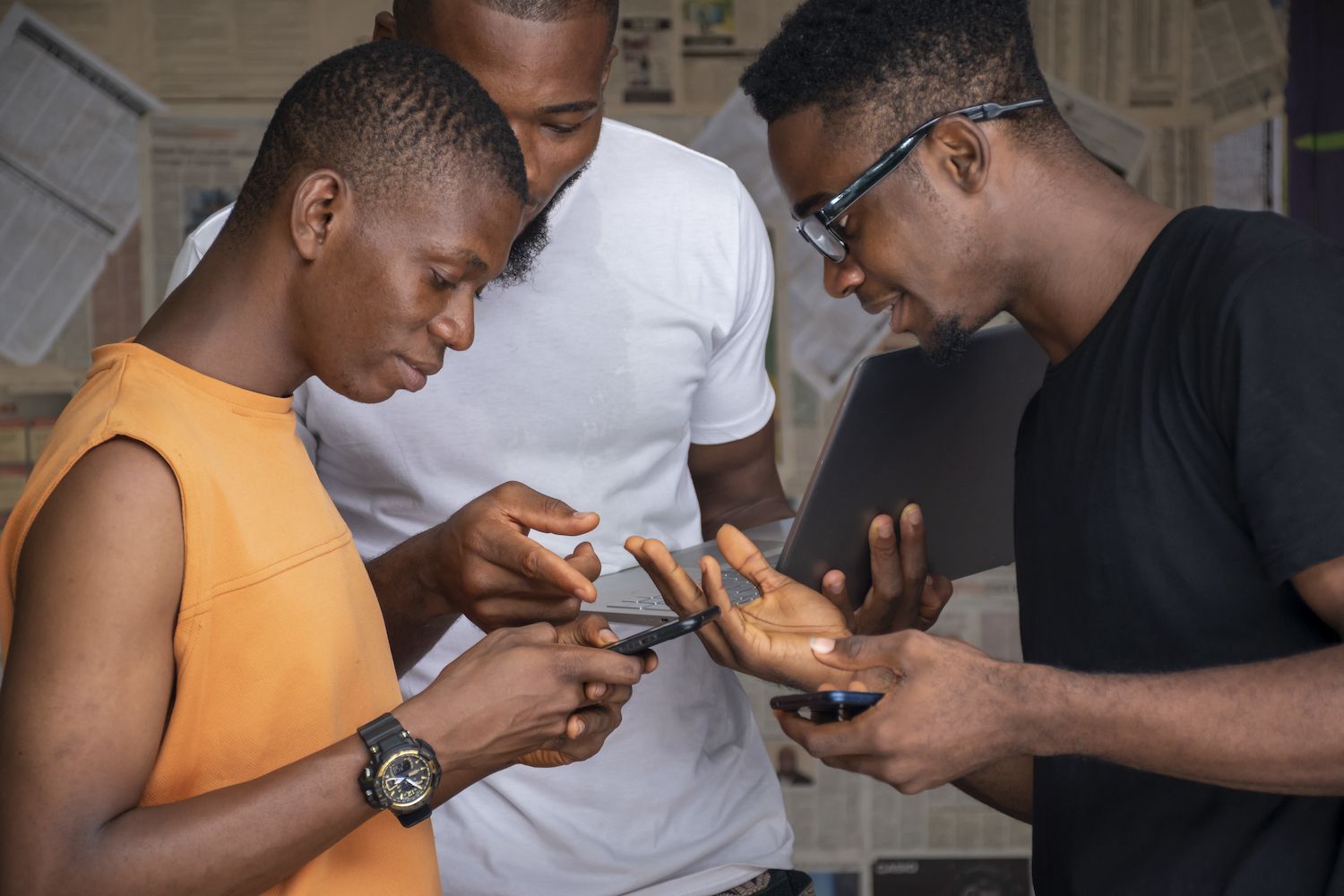
Make use of the token you obtained as a Bearer token and incorporate it into the Authorization header.
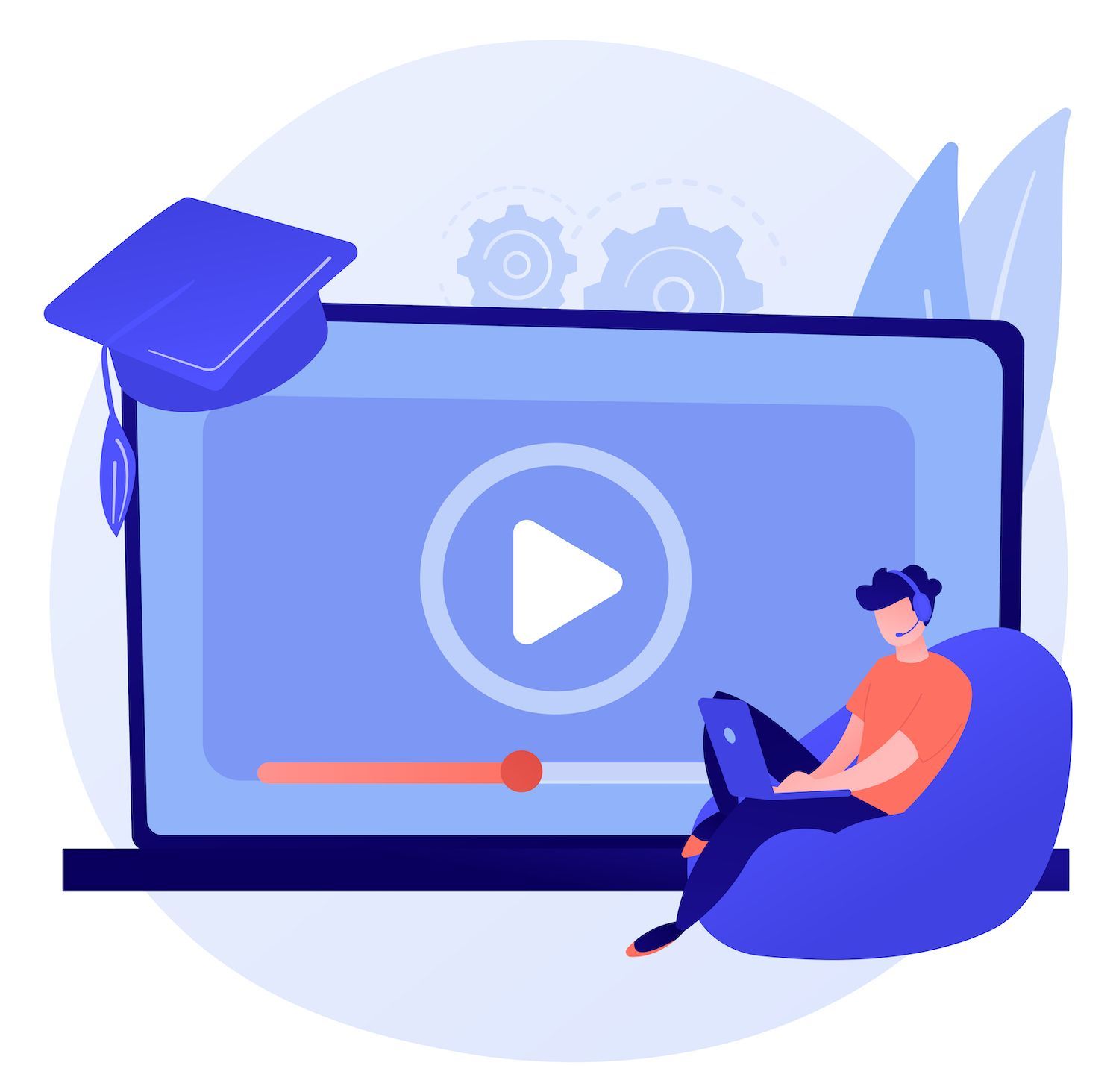
What can you do to fix API Errors
An error code by itself will not be enough. A message for error that can be easily read by a human is needed. Laravel has a wide range options to solve the issue. Utilize a fallback, catch block, or send a custom response. The following code you put in UserController UserController
shows this.
if (!$user || !Hash::check($request->password, $user->password)) return response([ 'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it easy to develop and test the API and test it. Mapping objects gives the ability to interact easily with databases.
In addition, it functions as a middleware, the token Sanctum by Laravel will help protect your APIs within a brief period of.
- The My dashboard is easy to manage and set up within My dashboard. My dashboard
- Support is available 24/7.
- The most reliable Google Cloud Platform hardware and network powered by Kubernetes to maximize scalability
- Top-of-the-line Cloudflare integration to speed up the process and enhance security
- Global reach through more than 35 data centers as well as more than 275 points of presence all over the globe
This article first appeared on this website
Article was posted on here