It is the procedure of building GraphQL APIs that make the use of Node
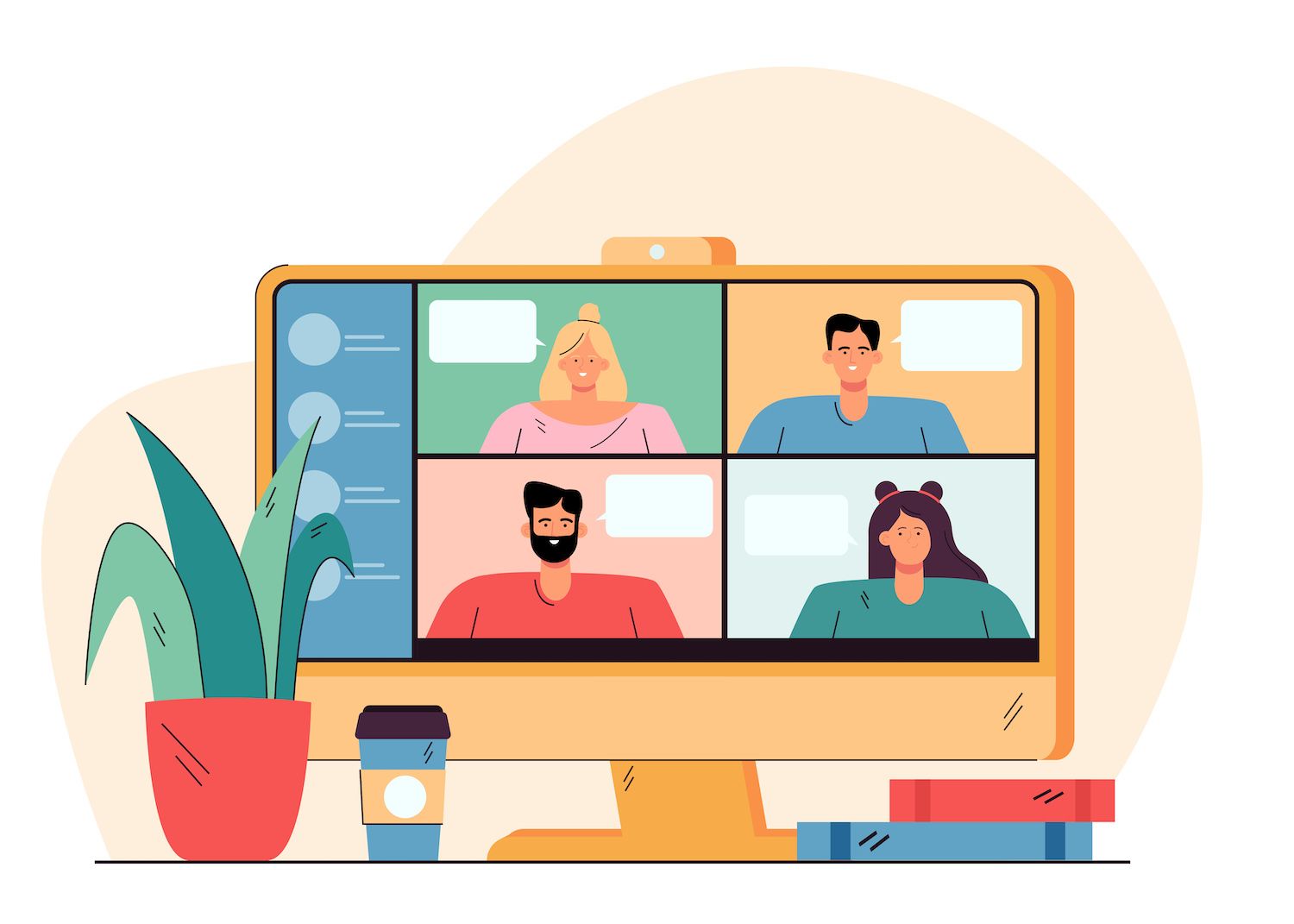
GraphQL is among the most sophisticated technology used to create APIs. Though RESTful APIs may be the preferred method commonly used to provide information to applications however they do have their drawbacks and limitations, which GraphQL is attempting to overcome.
GraphQL is the query language created by Facebook and made an open source project in the year of 2015. T offers a simple and flexible syntax which can be used to define the information that is accessible through an API.
What do you actually mean by GraphQL?
The official website: "GraphQL is a API query language, as well for an API runtime, which is able to assist in creating queries from the data you already have. GraphQL gives a full and easy explanation of the information you provide via your API. Users can obtain all the data they need and no less. It makes it simple to create APIs in shorter amount of time and gives developers the ability to make use of robust tools."
To build an GraphQL service, start by being able to define schema types, before creating fields to match these types of. Then, you create the function resolver to run on each type and field at the time that information is required to be collected by the client's side.
The GraphQL Terminology
GraphQL type system can be used to determine what information is available to request and what data can be altered. This is at the heart of GraphQL. There are a variety of ways of explaining and changing the information within the design of GraphQ.
Types
GraphQL objects include fields with elements of solid. It is advised to construct 1-1 mappings between models and GraphQL types. Here's an illustration GraphQL Type:
You Type User, Type Your username! "! "!" is the name first that's required. Name: username: String todos"[Todo"[Todo"[Todo]" an entirely distinct GraphQL type
Queries
GraphQL queries define all queries users can use in order to connect to the GraphQL API. Users should construct the initial querythat encompasses all currently being queries that use the protocol.
It's then it is possible to find the query, and match it with the RESTful API that has been validated and compatible with the API:
Typ RootQuery user(id ID) User # is matched with API/IDUsers. The # is API/users todostodo(id ID! ): Todo # Corresponds to GET /api/todos/:id todos: [Todo] # Corresponds to GET /api/todos
Mutations
If GraphQL is among the GET requests and the modifications are POST PUT, Patch as well as the DELETE request which modify the GraphQL API.
Every change is incorporated to create an RootMutation to show:
type RootMutation *
There is a chance that you've already utilized the input kinds to indicate the kinds of transformations that could be performed like users' input, TodoInput. It's a great idea to determine the input type before changing or creating the original source.
There is a way to explain thenput kinds like:
Input UserInput: Firstname String! String for email with lastname! username: String!
Resolvers
Create a fresh resolvers.js file and add the following code to it:
import sequelize from '../models'; export default function resolvers () const models = sequelize.models; return // Resolvers for Queries RootQuery: user (root, id , context) return models.User.findById(id, context); , users (root, args, context) return models.User.findAll(, context); , User: todos (user) return user.getTodos(); , // Resolvers for Mutations RootMutation: createUser (root, input , context) return models.User.create(input, context); , updateUser (root, id, input , context) return models.User.update(input, ...context, where: id ); , removeUser (root, id , context) return models.User.destroy(input, ...context, where: id ); , // ... Resolvers for Todos go here ; }
Schema
GraphQL schema defines the data GraphQL provides to the public at large. This means that all kinds of queries, kinds, as well as modifications, are covered in the schema and accessible to everyone.
These are the steps to make public the types of questions you ask, as well as other changes that are available to the public
schema query: RootQuery mutation: RootMutation
The script we've previously written, was enriched with the addition to it RootQuery that comes in well as RootMutation which was originally created to make them available across the globe.
What is GraphQL Do? Work With Nodejs and Expressjs
GraphQL is a programming language that is compatible with all programming languages and Node.js isn't among the languages. Its official GraphQL site has an article that describes JavaScript execution as well as the different variations of GraphQL that make the writing process using GraphQL straightforward.
GraphQL Apollo is a software that works with Node.js as well as Express.js as well as a way for users to start learning about GraphQL.
The course will teach students how to develop and build your first GraphQL application using Nodes.js along with Express.js, the Express.js backend framework, which integrates GraphQL Apollo. The course will be covered in the next section.
Configuring GraphQL By using Express.js
The procedure of creating the GraphQL API server with Express.js is a great way for beginners to get started. In this article we'll explain the steps to create an GraphQL server.
Create a new project with Express
Create an account within your project. Then, install Express.js via the following instructions:
cd && npm init -y npm installation express
The command will create a fresh package.json file and it's installed using the Express.js library within the application which you're using.
Are you interested in learning how we've grown the number of our visitors by one thousand?
Join over 20,000 other users who get our email each week. Our emails are filled with exclusive WordPress specifics!
Our next step is to arrange our activities in manner like the image below. The program is composed of various modules to accomplish its tasks, like the user's task, for example.
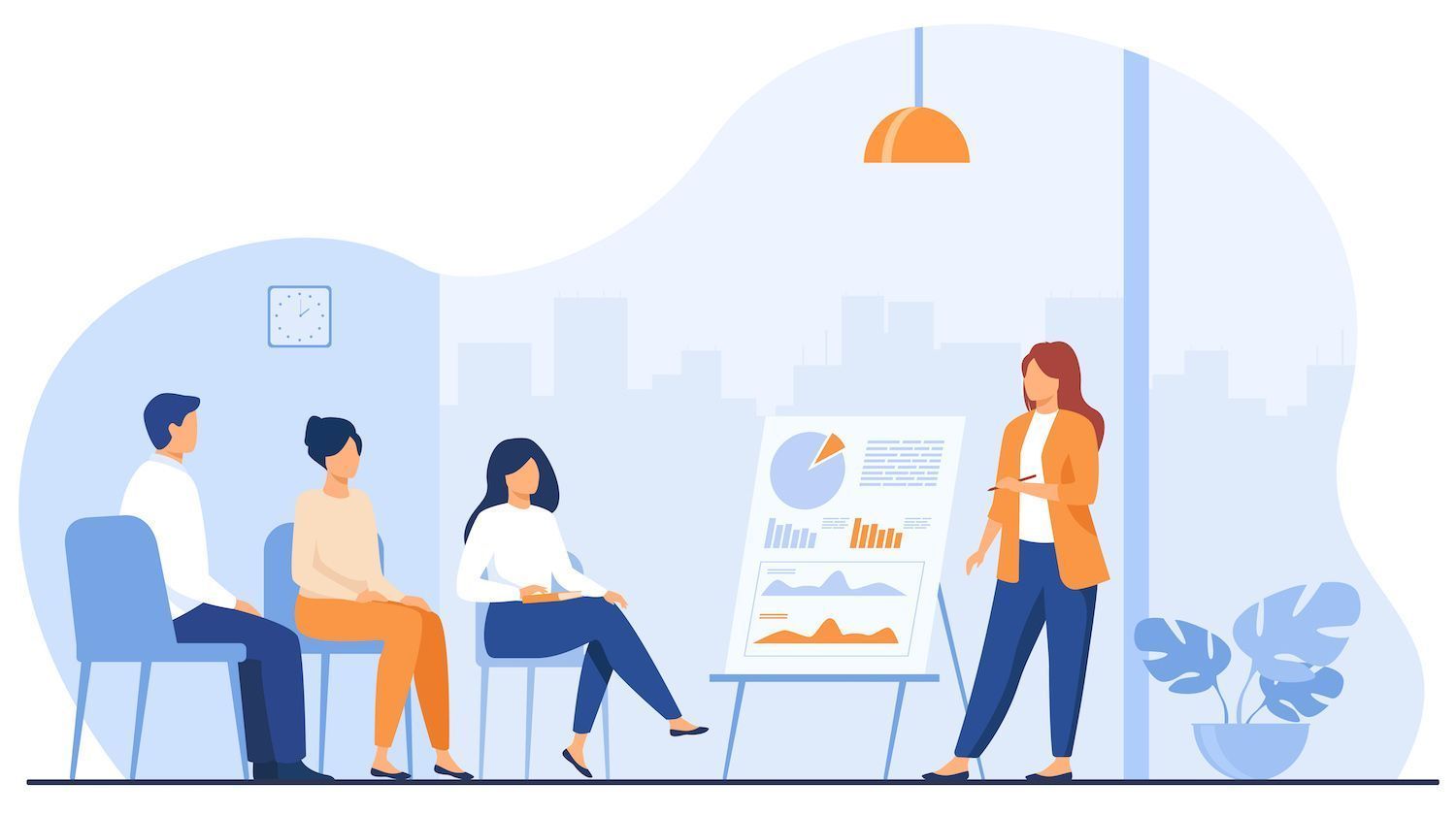
Initialize GraphQL
Set up your GraphQL Express.js dependency. The following command is needed to set up the installation
npm install apollo-server-express graphql @graphql-tools/schema --save
Method for making Schemas and Types
The following step is creating an index.jsfile. index.jsfile inside the directory that contains modules. This is the section of code:
const gql = require('apollo-server-express'); const users = require('./users'); const todos = require('./todos'); const GraphQLScalarType = require('graphql'); const makeExecutableSchema = require('@graphql-tools/schema'); const typeDefs = gql` scalar Time type Query getVersion: String! type Mutation version: String! `; const timeScalar = new GraphQLScalarType( name: 'Time', description: 'Time custom scalar type', serialize: (value) => value, ); const resolvers = Time: timeScalar, Query: getVersion: () => `v1`, , ; const schema = makeExecutableSchema( typeDefs: [typeDefs, users.typeDefs, todos.typeDefs], resolvers: [resolvers, users.resolvers, todos.resolvers], ); module.exports = schema;
Code Walkthrough
We'll take a closer look at this code snippet to see a more detailed look.
Step 1.
Then we download the required libraries, then we create the most popular types that include query and mutation. Both queries and mutations are an instance of GraphQL API as of now. We plan to extend the query and mutations in order to accommodate more schemas in the future.
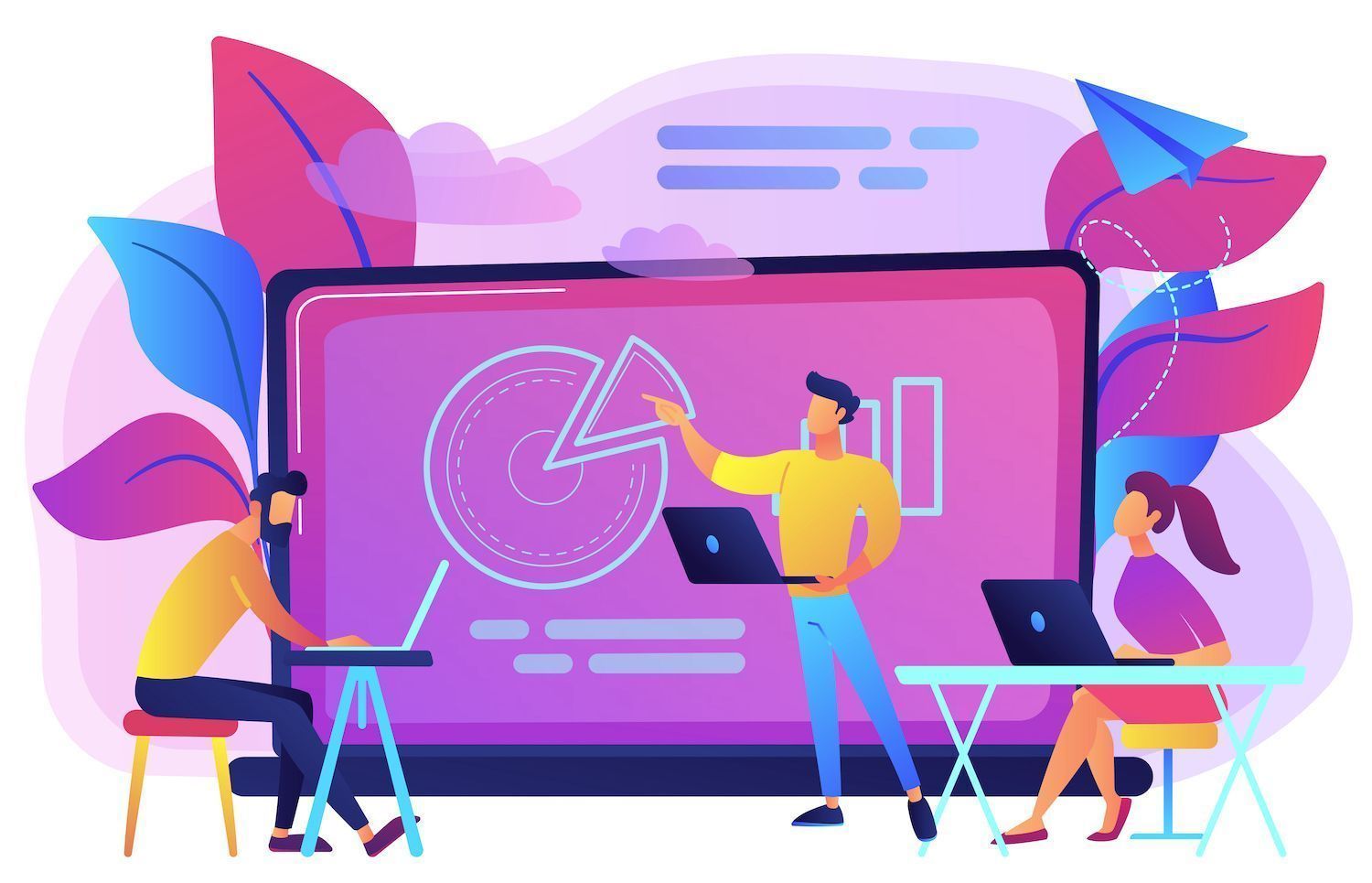
Step 2.
We then created the first type of scalar that can be defined as a representation of time and also our first resolver to the query, and the transformation we used before. Additionally, we generated a schema using this createExecutableEchema function.
The schema created includes schemas we've incorporated, and we will be developing additional schemas in the future as we develop them and eventually include them in the database.
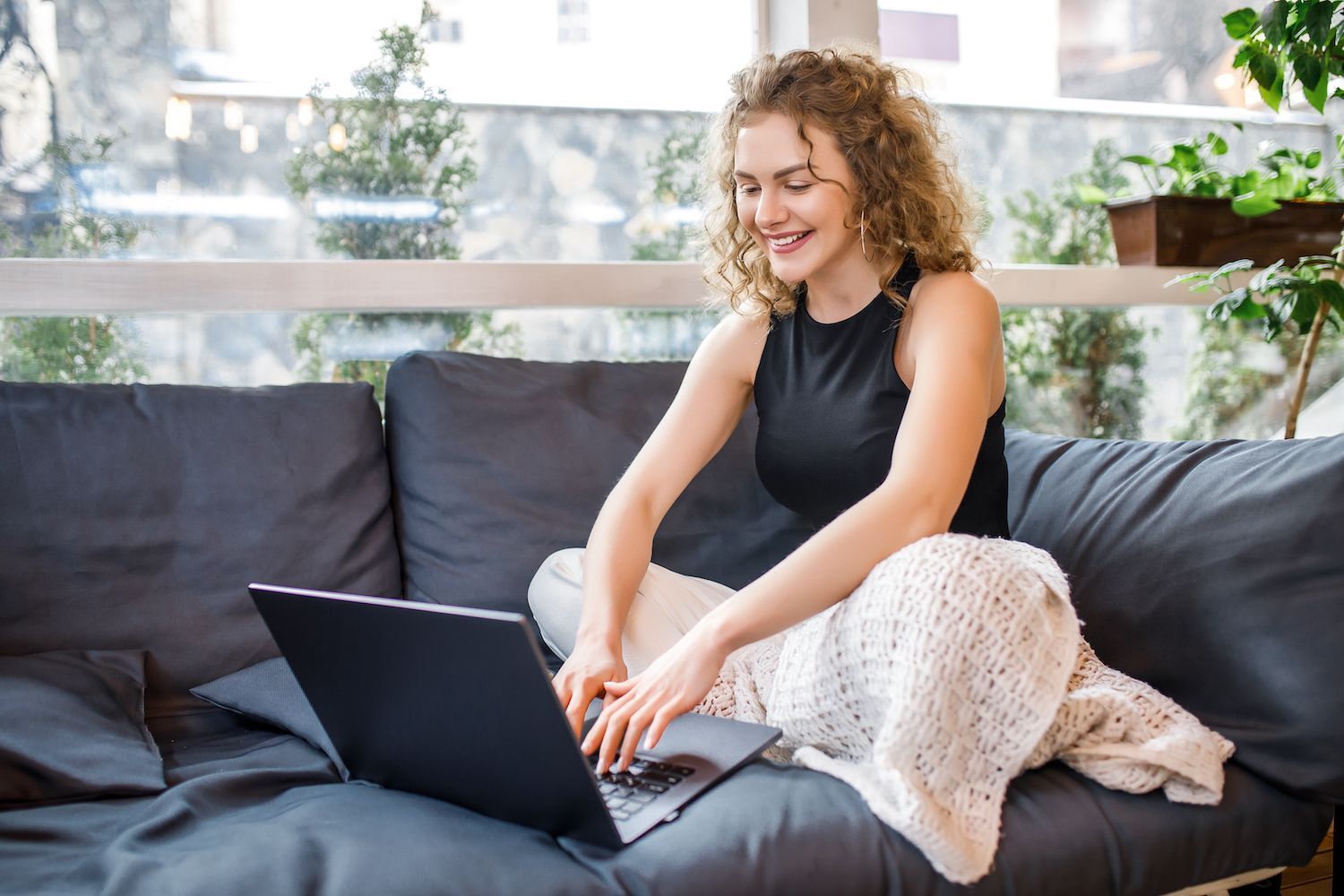
This code example shows how we have imported various schemas using the makeExecutableEchema method. This method helps to create an application that is organized to deal with the complicated issues. Next, we need to create our own schemas, which can be built upon Todo as well as the schemas for users that we've developed.
Creating Todo Schema
The Todo schema describes the basic tasks that users of the application can perform. The schema below performs Todo functions in addition to CRUD.
const gql = require('apollo-server-express'); const createTodo = require('./mutations/create-todo'); const updateTodo = require('./mutations/update-todo'); const removeTodo = require('./mutations/delete-todo'); const todo = require('./queries/todo'); const todos = require('./queries/todos'); const typeDefs = gql` type Todo id: ID! title: String description: String user: User input CreateTodoInput title: String! description: String isCompleted: Boolean input UpdateTodoInput title: String description: String isCompleted: Boolean *do; // Implement resolvers for the schema fieldsconst resolvers = Resolvers to resolve queriesQuery such as todo, todos and// Resolvers for Mutations Mutation: createTodo, updateTodo, removeTodo, , ; Module.exports = typeDefs;module.exports = typeDefs, resolvers ;
Code Walkthrough
Let's go through this code snippet. We'll break it down:
Step 1.
After that, we created the structure we needed to monitor our progress by using GraphQL form, input and expanding. The keywords extension extensions keyword lets you create additional queries or modify to your existing query, as well as the changes in the query that the change mentioned earlier caused.
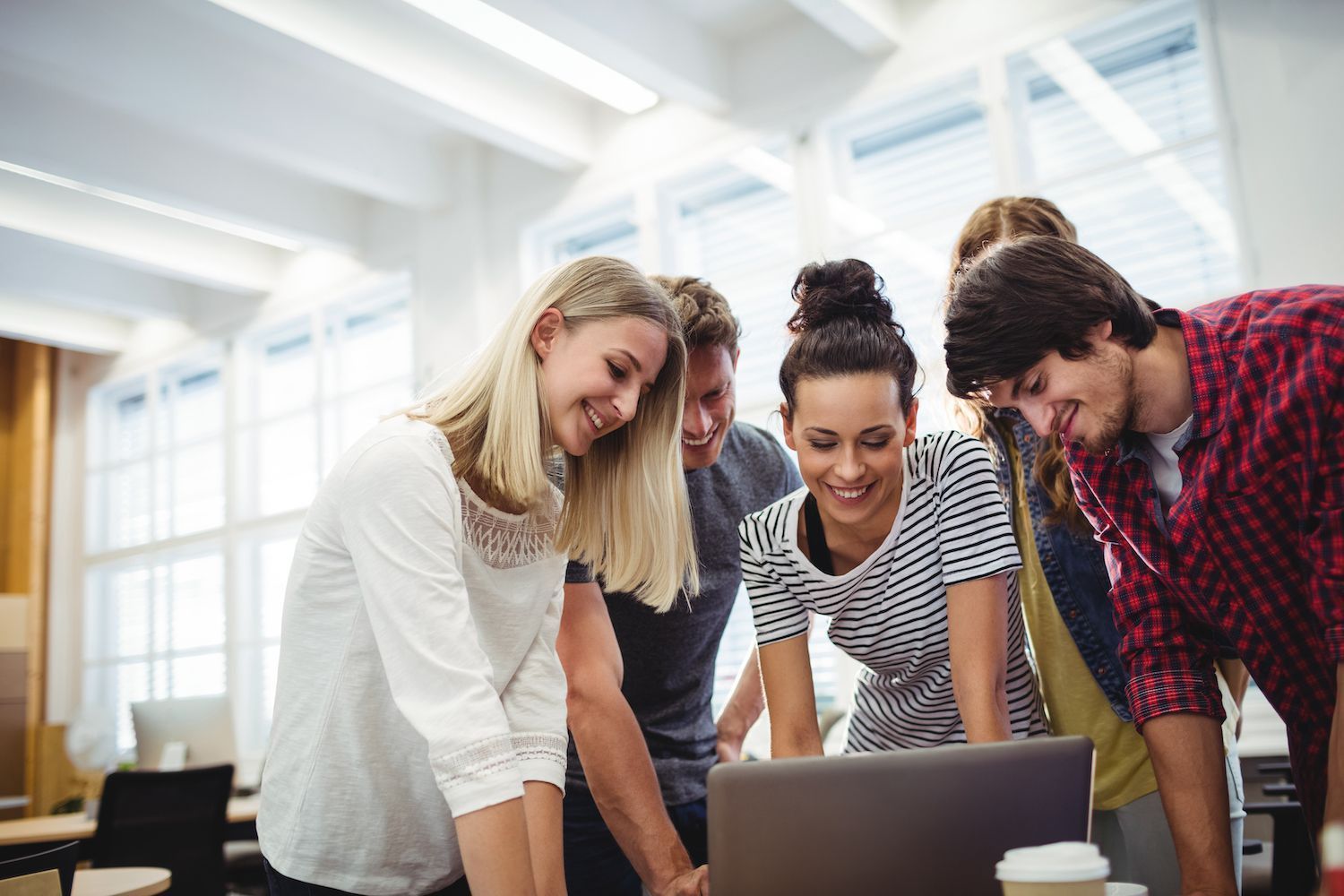
Step 2.
The resolver is one we designed, which will find the correct information when an issue, or a change is found to be.
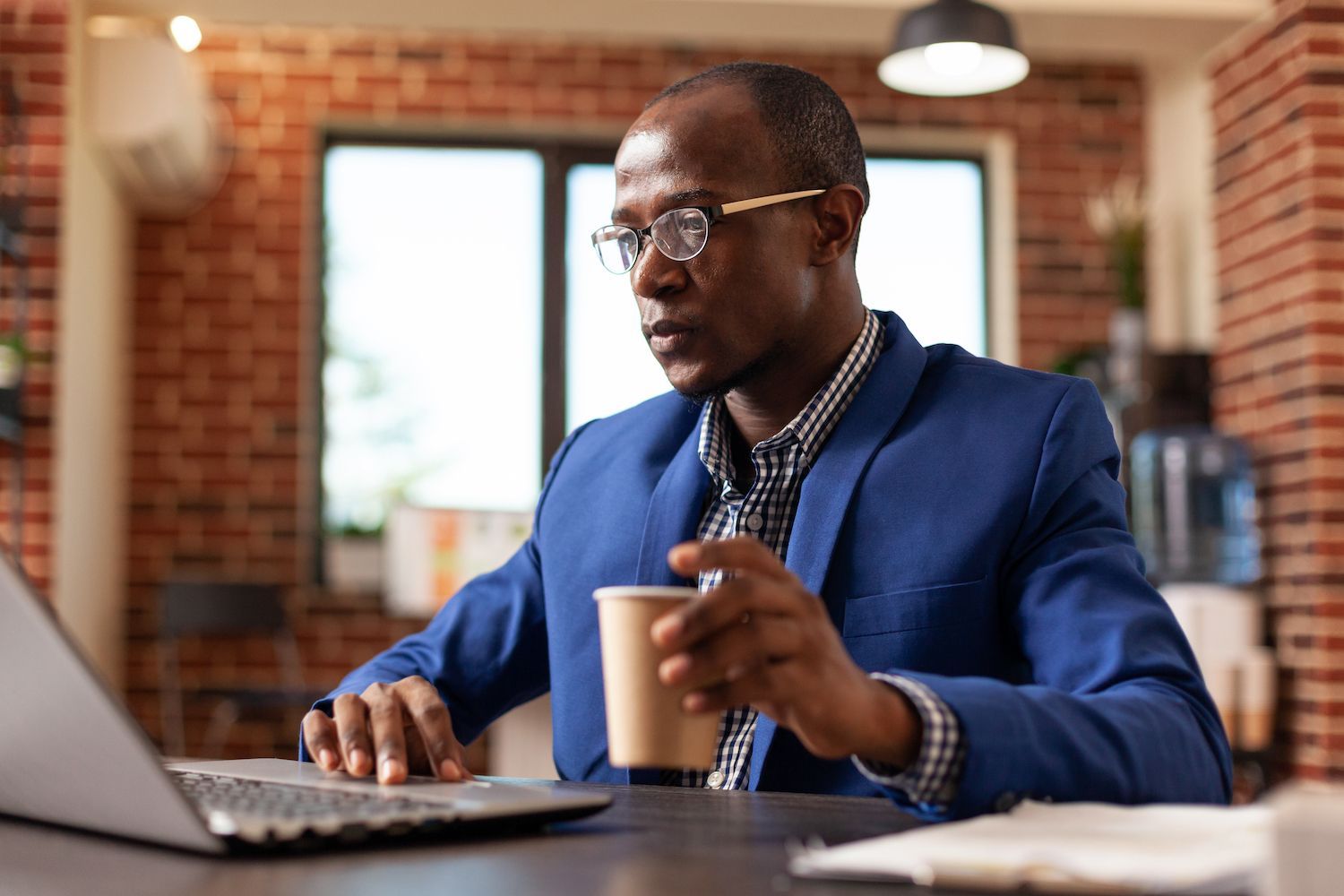
Utilizing resolver functions, it is possible to create specific strategies that control both the database and business logic like in the create-todo.js example.
Create an create-user.js file in the ./mutations
folder and connect the business logic to the form of a brand new Todo within your database.
const models = require('../../../models'); module.exports = async (root, input , context) => return models.todos.push( ...input ); ;
This code provides an easy method of creating a new Todo within our database using our ORM Sequelize. It is possible to learn more about Sequelize and how you can build it using Node.js.
You can use this same procedure to create different schemas based upon the requirements of your application. You can also upload the entire app into GitHub.
The next thing to do is to create a server with Express.js as well as to start the most recent Todo application using GraphQL along with Node.js
Configure, and then start the Server
Lastly, we will set up our server using the apollo-server-express library we install earlier and configure it.
The apollo-server-express is a simple wrapper of Apollo Server for Express.js, It's recommended because it has been developed to fit in Express.js development.
Using the examples we discussed above, let's configure the Express.js server to work with the newly installed apollo-server-express.
Make the server.js file in the root directory. Transfer your server.js file in the root directory. After that, copy the following code:
const express = require('express'); const ApolloServer = require('apollo-server-express'); const schema = require('./modules'); const app = express(); async function startServer() const server = new ApolloServer( schema ); await server.start(); server.applyMiddleware( app ); startServer(); app.listen( port: 3000 , () => console.log(`Server ready at http://localhost:3000`) );
The code you've observed above indicates that you've successfully completing the steps of creating your first CRUD GraphQL server for Todos and Users. You can start your development server and access the playground using http://localhost:3000/graphql. If everything goes as planned then you'll see the following display in the form of:
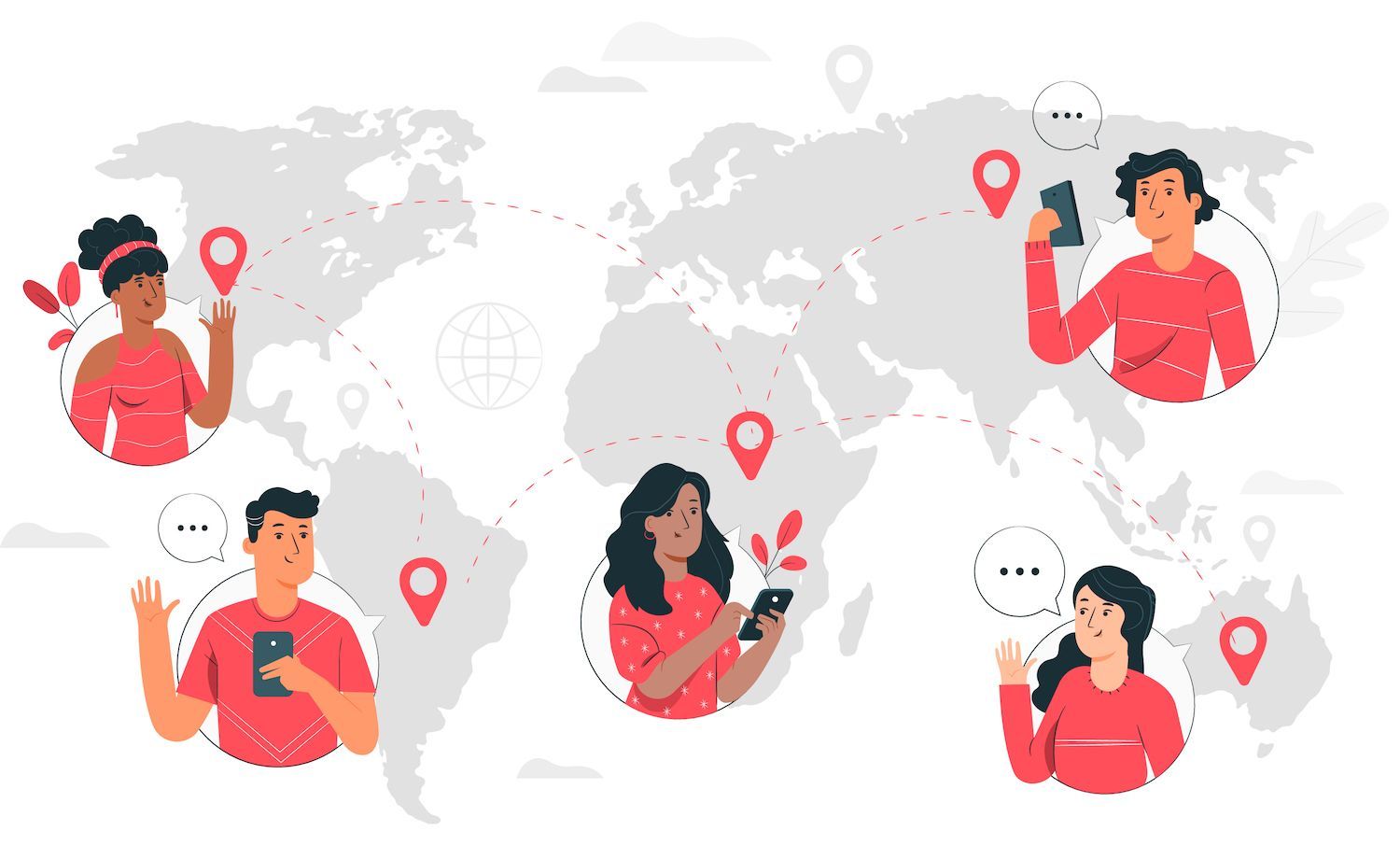
Summary
GraphQL is an advanced technology utilized by Facebook to aid in developing APIs that are large-scale with RESTful architectural design.
This article provides an overview of GraphQL and shows the steps to build your own customized GraphQL API with Express.js.
Let us know about your projects that you've developed using the help of GraphQL.
- Easy to set up and maintain My dashboard. My dashboard
- Support is accessible 24/7.
- The top Google Cloud Platform hardware and network is powered by Kubernetes for the highest capacity
- Premium Cloudflare integration to improve speed and also security
- Join the world's largest audience using more than 35 data centers as well as more than 275 POPS across the globe.
The original article appeared on on this website.
This article first appeared on on this website.
This article was originally posted this site.
This post was first seen on here