How can I add rate restrictions in an API of Laravel Application Laravel Application - (r)
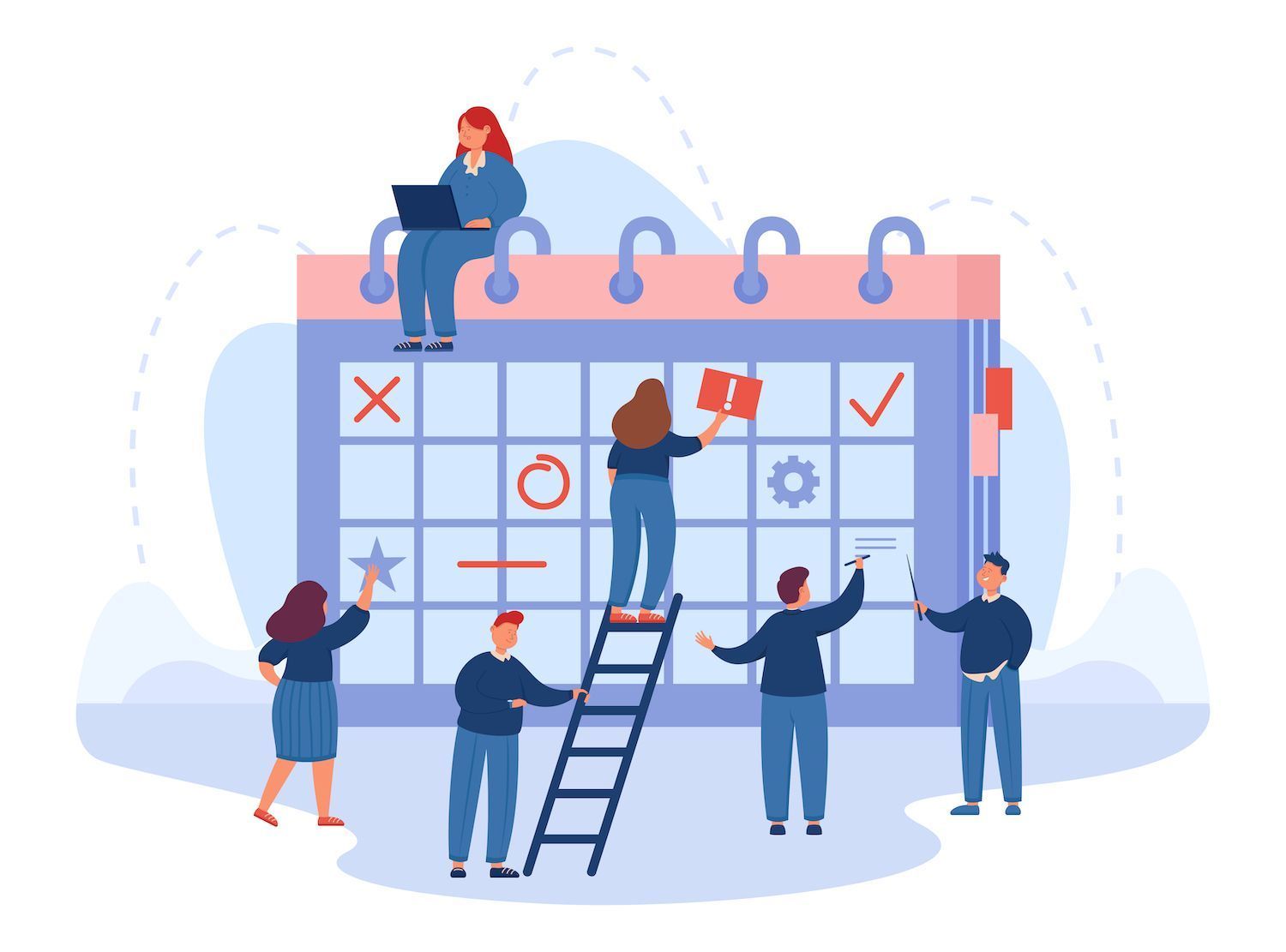
-sidebar-toc>
The restriction of usage is vital for protecting the resources of your app and website from misuse or excessive use. It could be because of an intentional human error, bot-driven attacks, or simply a misidentified attack, or a misuse of resources can limit the access that you've granted to your application and introduce severe vulnerabilities.
This article will provide methods to incorporate rates that limit in an API in Laravel. Laravel application.
Throttle Your API Traffic in Laravel
The principle of rate-limiting was to limit the use of an application's resources. There are a myriad of applications, but it's most effective for APIs that are accessible to the public on large, high-performance platforms. It ensures that all authorized users are able to gain access to all resources offered by the system.
Reducing the amount of data transfer is essential to ensure security in cost management as well as general security to ensure the stability of your system. This can prevent the occurrence of cyberattacks based upon request, like a distributed denial-of-service (DDoS) attacks. The type of attack used is repeatedly sending messages that overloads the system, and cause disruption to the web server or access to the web server.
There are a variety of approaches for setting limits on rates. You can use variables that identify the user to decide the person who has access to your app and when. Some common variables include:
- IP address Utilization of rates dependent on IP addresses allow users to limit the number of requests that can be processed per IP address. This could be especially useful in situations where the user is able to get access to an app without a login.
- API Key Limitations on access to API keys involves providing the user with an API key that's been generated, and setting limits on access per key basis. This technique lets you as well assign various degrees of access for the created API keys.
- Client ID It's possible to make a Client ID that users can embed in the body or header of an API requests. You can define the ID the access level, making sure that no one client will have the capability of monopolizing the resources that are available to it.
Laravel Middleware
How do you best to implement rate limits?
This instructional uses an API for mini libraries that is built upon the Laravel 10 framework to demonstrate the use of Laravel Throttle. The sample starter project contains the essential create read, update and remove (CRUD) implementations required to manage books in the range. It also includes two other methods of demonstrating specific limitations on the rates.
The conditions
This tutorial assumes you're familiar with the basic concepts of API development using Laravel. You must have the following abilities:
- PHP 8.2, Composer, as well as Laravel is installed and set-up locally on the device you are using.
- A user account for GitHub, GitLab and Bitbucket to push your application
Additionally, you can use your My tool to create and launch the API. Utilize the design template before looking at what happens to the whole program.
Laravel Application Installation
- At the start, copy the layout template..
- Create a .env file in the root directory of your project and replicate the content of .env.example into it.
- Once you've finished, make sure to complete your installation using the steps required to install app dependencies, and then create an application key.
composer install php artisan key:generate
If the command isn't able to immediately add app keys to your .env file, execute the php artisan key:generate command with --show
after which take the key generated and then add it to the .env file as the key value within the app key file
.
- Once the dependencies installation and the key generation for application has been completed, you can open your application by selecting the following option
php artisan serve
This command starts the application and makes it accessible via the browser at https://127.0.0.1:8000
.
- Go to the URL and ensure that the Laravel welcome page appears:
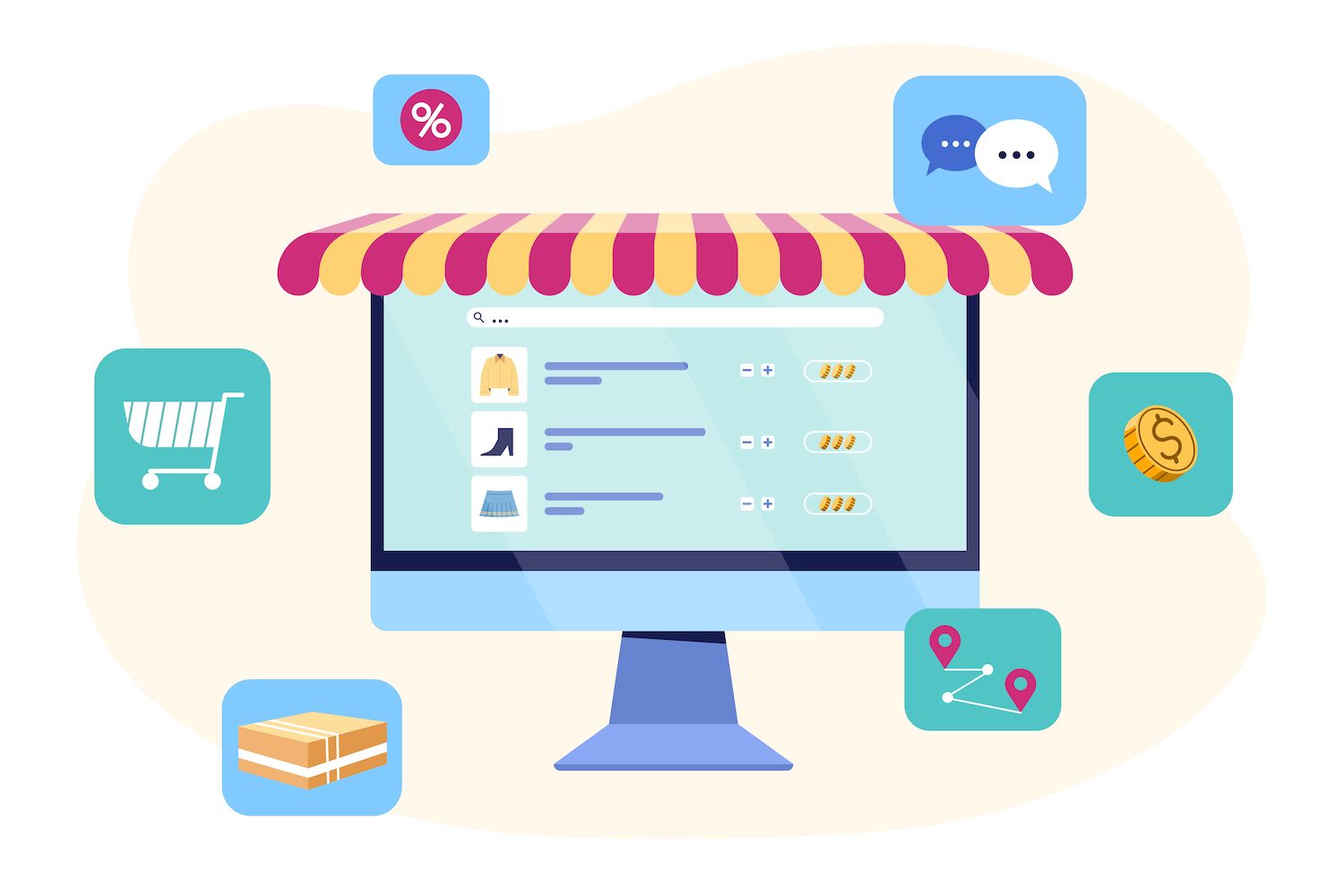
Configuring Databases
Create and configure the database to allow application into My.
- Log in to My Account. Go to the My Account dashboard, then choose Add service. "Add service" button:
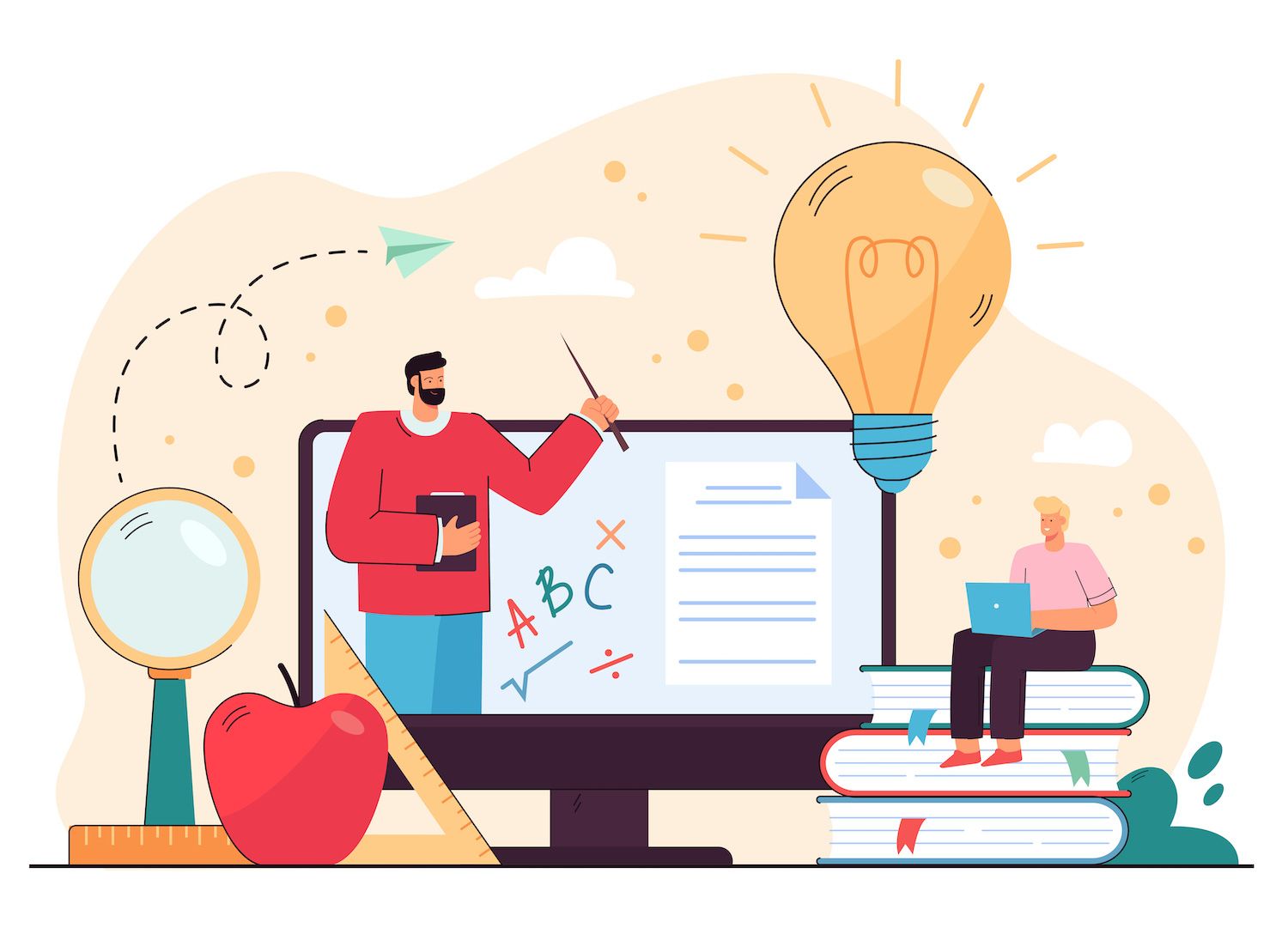
- In the Add Service listing Click "Database" and set the parameters that will start your database instance:
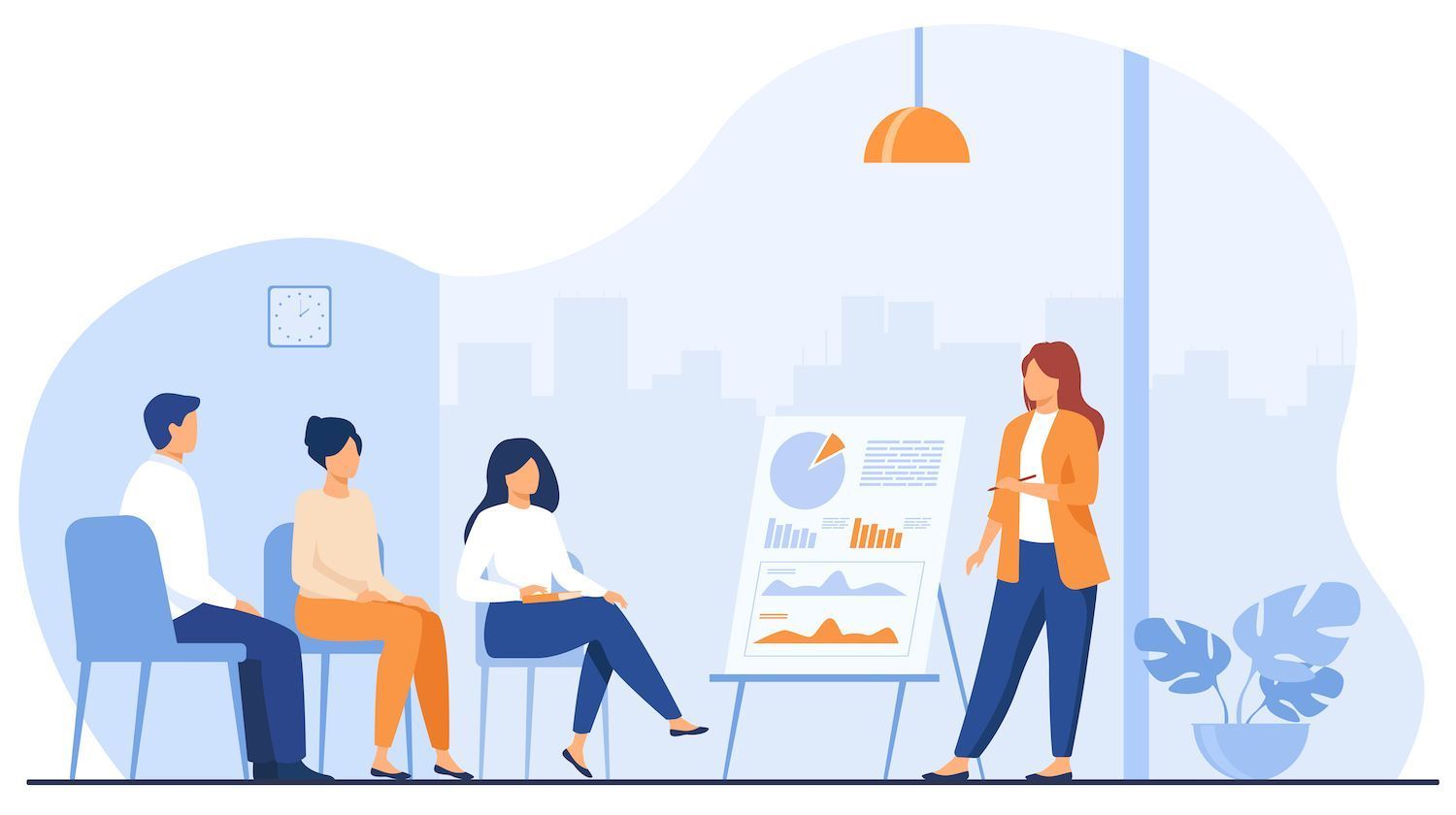
This tutorial uses MariaDB but you could also select one of the other supported Laravel databases, which provide.
- After you've finished your database's information After that, you can click the Proceed button to finalize the procedure.
The databases that are provisioned have connections to internal and external. Best practice is to connect internal parameters for applications running on the same account. Additionally, you can connect external connections parameters permit you to connect to externally. So, use the credentials from an external database in your application.
- Transfer and edit the app's database .env credentials with external credentials, as illustrated by the following image:
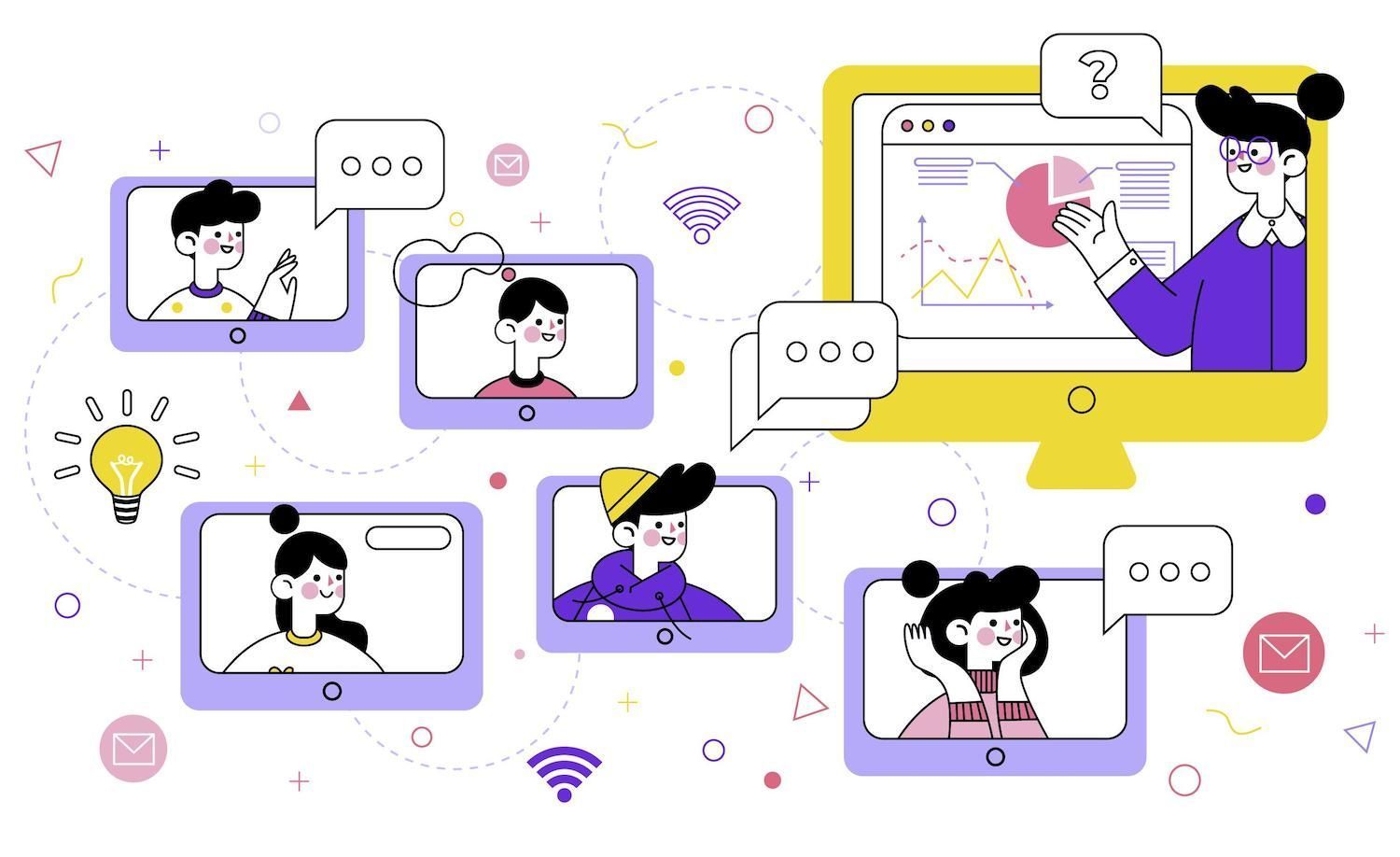
DB_CONNECTION=mysql DB_HOST=your_host_name DB_PORT=your_port DB_DATABASE=your_database_info DB_USERNAME=your_username DB_PASSWORD=your_password
- Once you've added the password to the database, check your connection with Database migration by using these commands:
php artisan migrate
If all functions are working correctly You should see exactly the same result as in below.
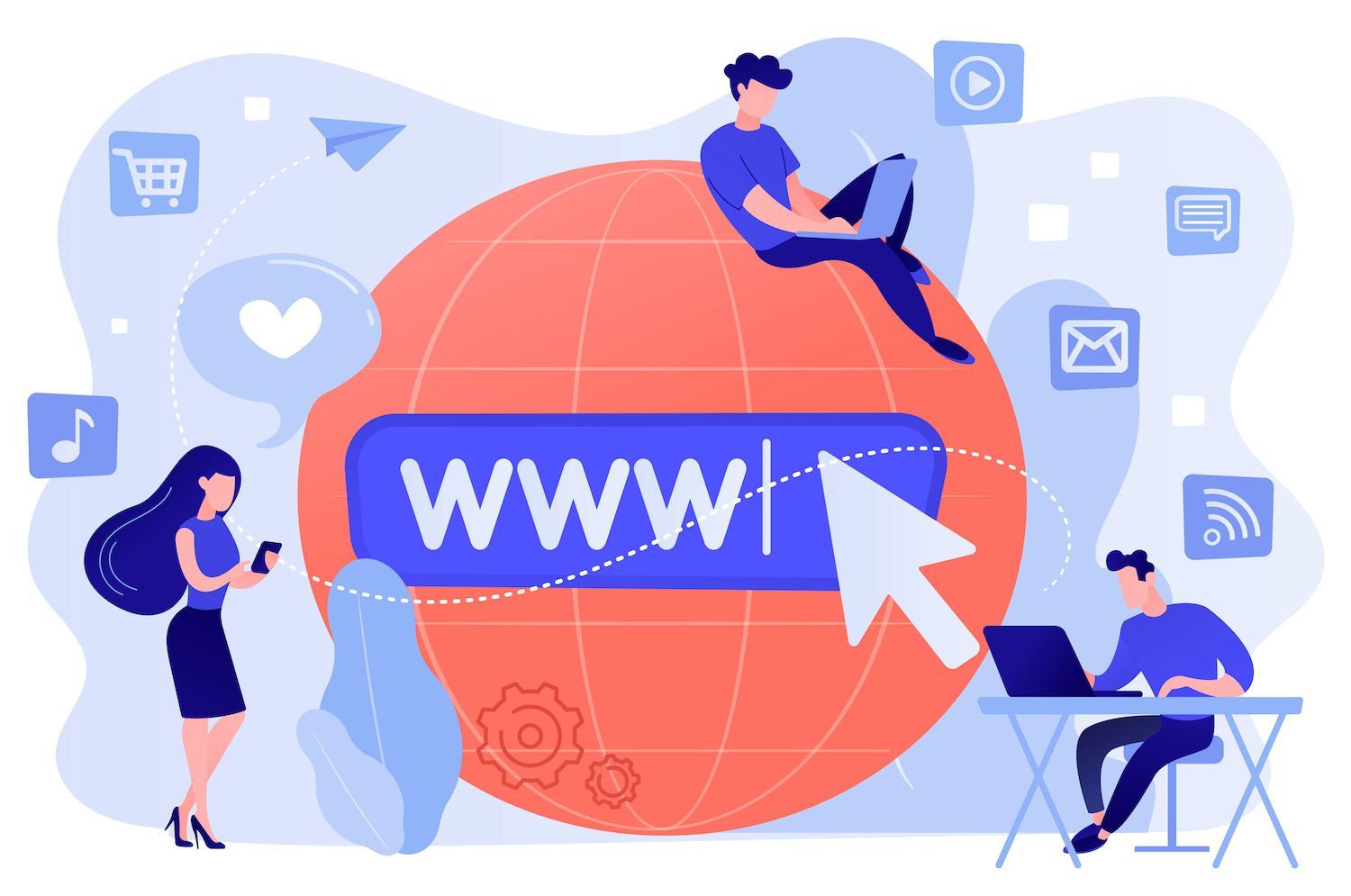
- After that, choose the next option to display every route available. There are also routes which have been developed.
php artisan route:list
It's now possible to access the API to access the following ends:
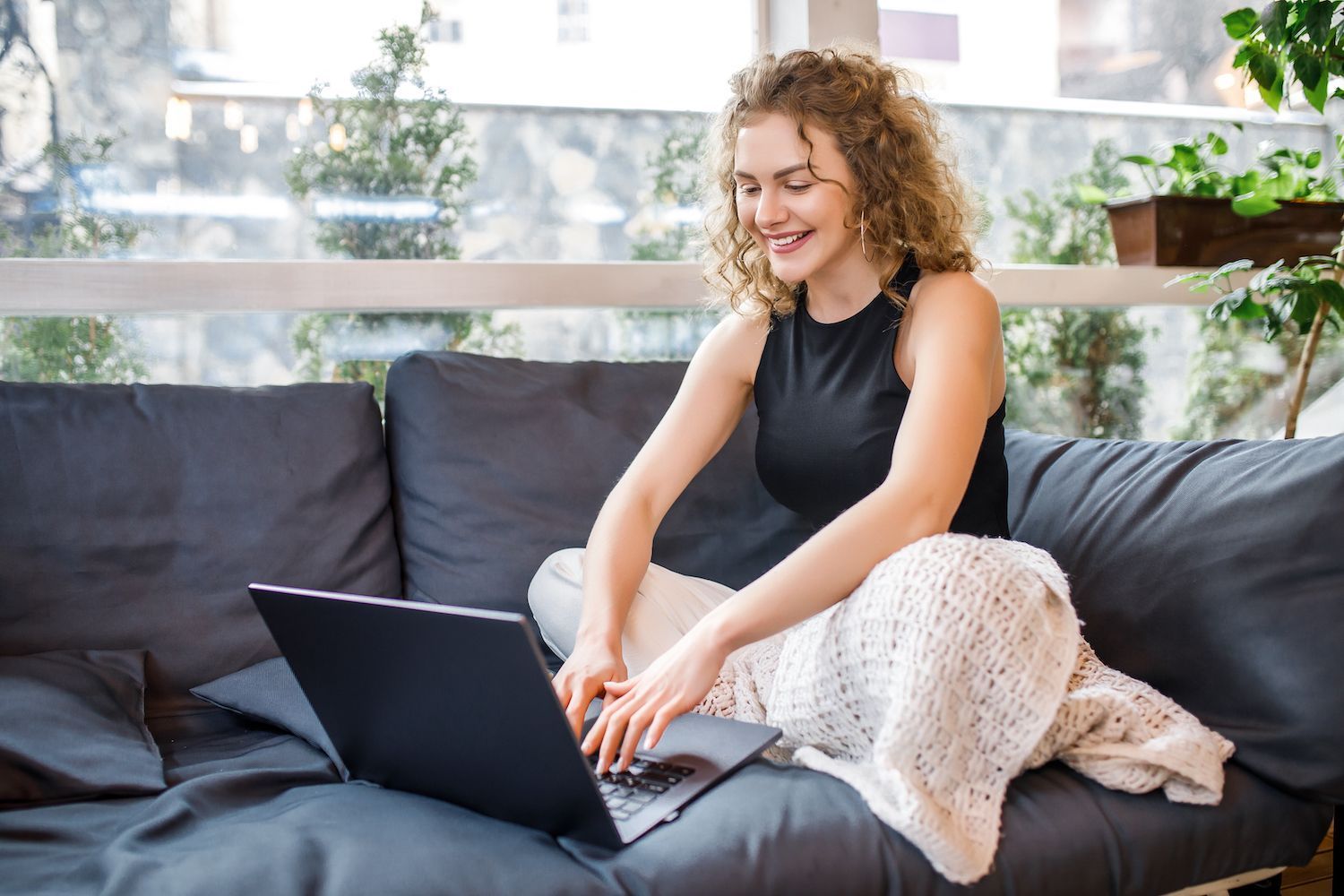
- Start the application and confirm that everything is still working. Verify these endspoints by using an application that runs on terminals, such as Postman or CURL.
How do you best to limit your rate within the Laravel Application
- Installation of the Laravel Throttle module using the instructions below:
composer require "graham-campbell/throttle:^10.0"
- Also, it is possible to alter the Laravel Throttle configurations through the publishing in the
vendor configuration
file.
php artisan vendor:publish --provider="GrahamCampbell\Throttle\ThrottleServiceProvider"
What can you do to block IP addresses?
Another way to limit rate is that you restrict access to an IP group of addresses.
- In the beginning you will have to develop the middleware required:
php artisan make:middleware RestrictMiddleware
- Next, open the created app/Http/Middleware/RestrictMiddleware.php middleware file and replace the code in the
handle
function with the snippet below. Be sure to addthe Use app
as one of the imports at the top of your middleware file.
$restrictedIps = ['127.0.0.1', '102.129.158.0']; if(in_array($request->ip(), $restrictedIps)) App::abort(403, 'Request forbidden'); return $next($request);
- In app/Http/Kernel.php app/Http/Kernel.php Create an Middleware alias by updating to the
middlewareAliases
array, as the following:
protected $middlewareAliases = [ . . . 'custom.restrict' => \App\Http\Middleware\RestrictMiddleware::class, ];
- After that, you can apply the middleware on your
restricted route
on by the routes/api.php file as you would like and test:
Route::middleware(['custom.restrict'])->group(function () Route::get('/restricted-route', [BookController::class, 'getBooks']); );
If it is working properly the middleware will block all requests from IP addresses that are within the array called the $restrictedIps
array. This is inclusive of 127.0.0.1
and 102.129.158.0
. If you attempt to connect these IP addresses, they'll give an error code of 403 rejected response. Here is an illustration of this:
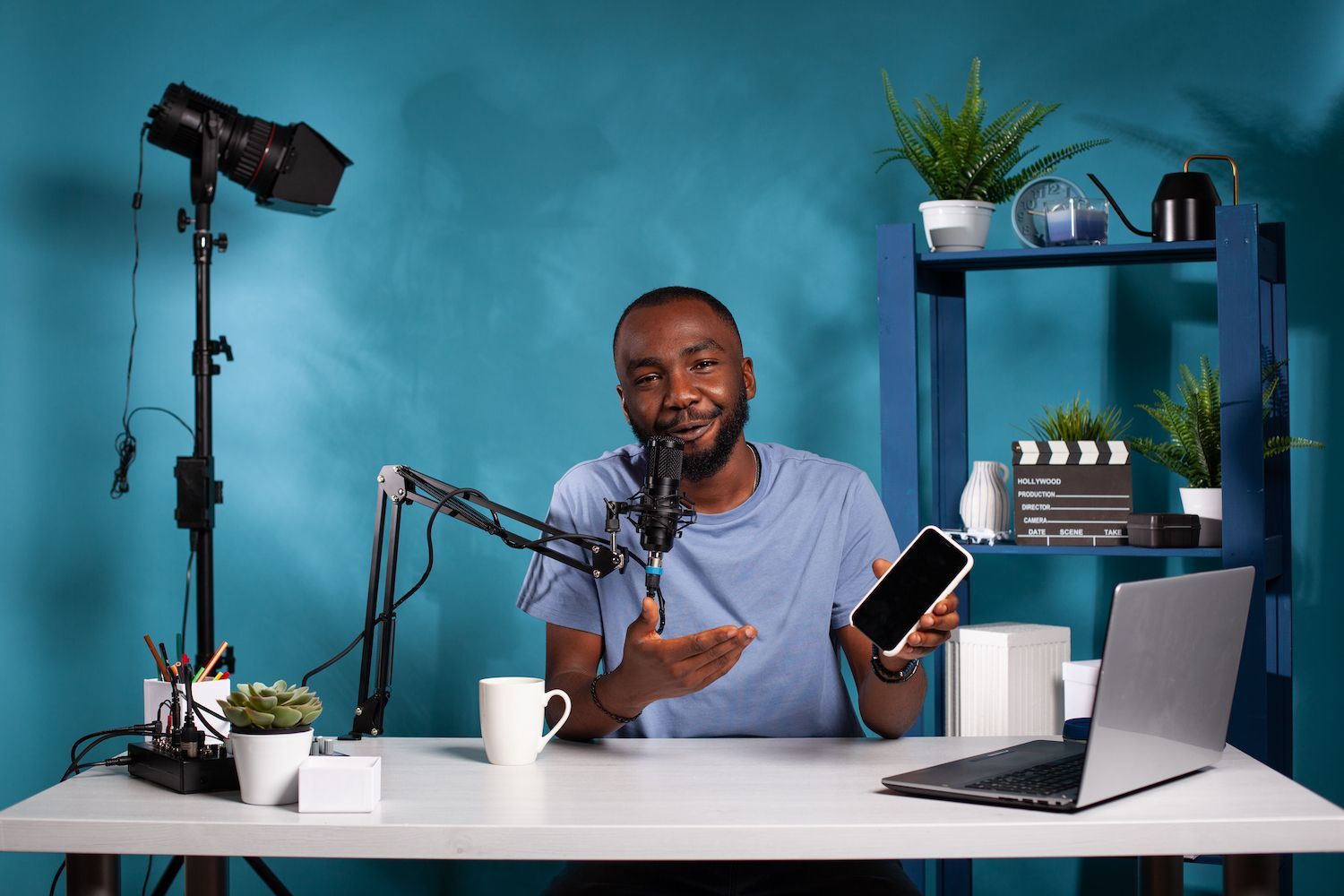
How can I throttle requests through IP address
In the next step, you'll give each request a rating based on your IP address.
- Set up this Throttle middleware on
book
connectivity'sPATCH
ANDPATCH
routes. routes/api.php:
Route::middleware(['throttle:minute'])->group(function () Route::get('/book', [BookController::class, 'getBooks']); ); Route::middleware(['throttle:5,1'])->group(function () Route::patch('/book', [BookController::class, 'updateBook']); );
- You must also update the
configureRateLimiting
function in the app/Providers/RouteServiceProvider file with the middleware you added to the above routes.
... RateLimiter::for('minute', function (Request $request) return Limit::perMinute(5)->by($request->ip()); );
The limit for requests is established for the book. The book
ends at five minutes. The illustration of this is in the next.
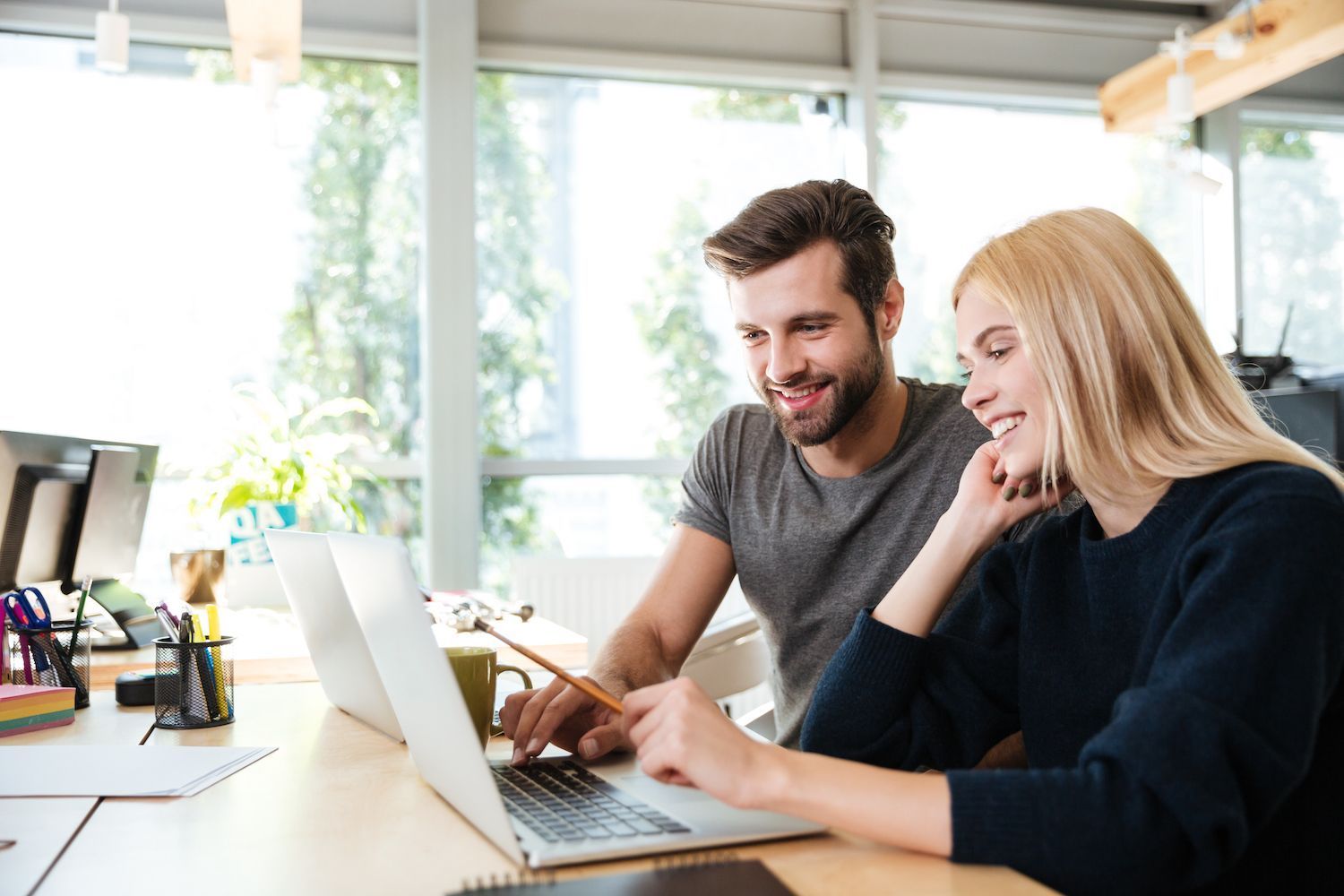
What can be throttled depending on the User ID as well as Sessions
- To rate limit using
user_id
andsession
parameters, update theconfigureRateLimiting
function in the app/Providers/RouteServiceProvider file with the following additional limiters and variables:
... RateLimiter::for('user', function (Request $request) return Limit::perMinute(10)->by($request->user()?->id ? : $request->ip()); ); RateLimiter::for('session', function (Request $request) return Limit::perMinute(15)->by($request->session()->get('key') ? : $request->ip()); );
- After that, you could apply this code to
the ID/book/GET
in addition tothe book POST
routes from routes/api.php:routes/api.php
file:
Route::middleware(['throttle:user'])->group(function () Route::get('/book/id', [BookController::class, 'getBook']); ); Route::middleware(['throttle:session'])->group(function () Route::post('/book', [BookController::class, 'createBook']); );
The limit code is only applicable to requests that use the user_id
as well as sessions session
and session.
Alternative Methods for Throttling
Laravel Throttle features several other techniques to provide greater control over the rate-limiting apps you use. The methods are:
attempt
hits the limit it reaches increasing the number of hits and returns a boolean that indicates whether the maximum number of hits has been reached.hit
hit -- hits the Throttle and increases the amount of hits. It then returnsthe number
that allows another (optional) method of calling.clear
resets the throttle value up to zero. This then returnsto $this
to call again, if you'd like to.- count The count provides the total number of hits to the Throttle.
test
gives an object that shows whether or not the Throttle hitting limit was exceeded.
- In order to test the effectiveness of rate-limiting techniques, you can create a middleware application named CustomMiddleware by using the following command:
php artisan make:middleware CustomMiddleware
- Then, add the following import files to the newly created middleware file in app/Http/Middleware/CustomMiddleware.php:
use GrahamCampbell\Throttle\Facades\Throttle; use App;
- After that, change the handle method's contents with the code below: Replace
the handle
method by using this code sample
$throttler = Throttle::get($request, 5, 1); Throttle::attempt($request); if(!$throttler->check()) App::abort(429, 'Too many requests'); return $next($request);
- Within in the app/Http/Kernel.php file, create an alias for the middleware application by altering the middlewareAliases array.
middlewareAliases
array in the following method.
protected $middlewareAliases = [ . . . 'custom.throttle' => \App\Http\Middleware\CustomMiddleware::class, ];
- If you do, then you're able to use this middleware in your
route that you have customized
inside your routes/api.php file:
Route::middleware(['custom.throttle'])->group(function () Route::get('/custom-route', [BookController::class, 'getBooks']); );
The custom middleware that was just recently put in place will be able to find out if the limit for throttle was exceeded employing the check/code technique. If the limit is overridden, it will give a 429 error. In other cases this will permit the request to continue.
What is the best way to have the application installed on the Server
Once you've been able to set up rate limits for the Laravel application, make sure you deploy your application to the server in order that it is accessible all over the world.
- On your dashboard, select From your dashboard the "Add Services" button, and then click the program in the menu. Join to your Git account to your existing account and choose the right repository to deploy.
- Within the Basic Info, name your application and select the best location to host your data center. Be sure to add the required environment variables for your application. These are the same as that you have in your own .env file: app_key,
app_key
along with the database configuration variables.
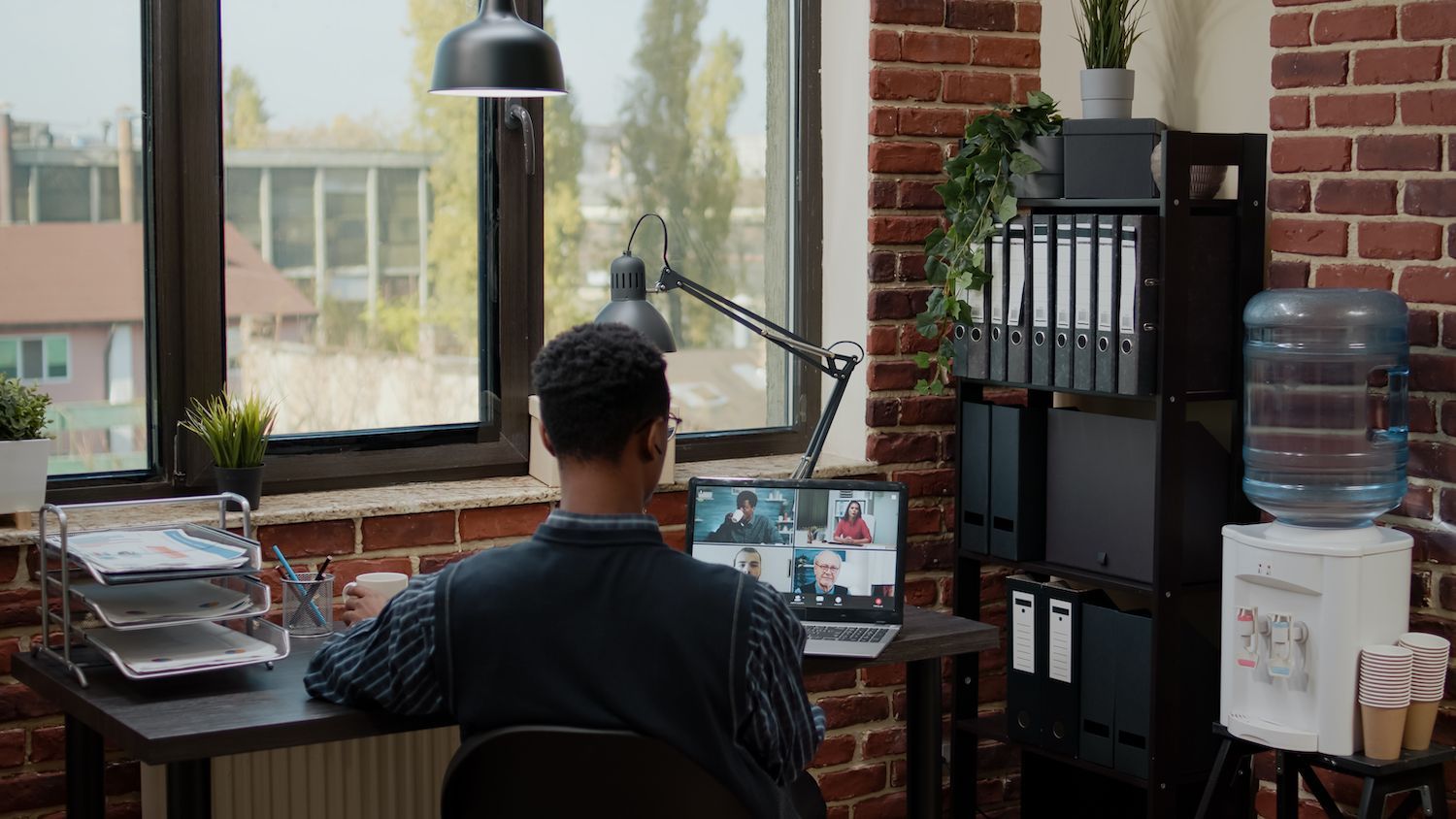
- Use The Keep button to select the variables used to construct your environment. You can leave your defaults since the auto-fill function fills every required parameters.
- In the process tab is an choice to change the default values or choose a different name for the process. It is also possible to select the pod or instance sizes in the tab.
- At the end of the process, the tab for payment tab offers a listing of options. Choose the payment method you'd prefer to utilize for the payment.
- After you've completed that, head to and select the App tab to view the application list that are currently in use.
- Click on the name of the app to find more information on its use such as the one shown below. You can use the URL to open the application.
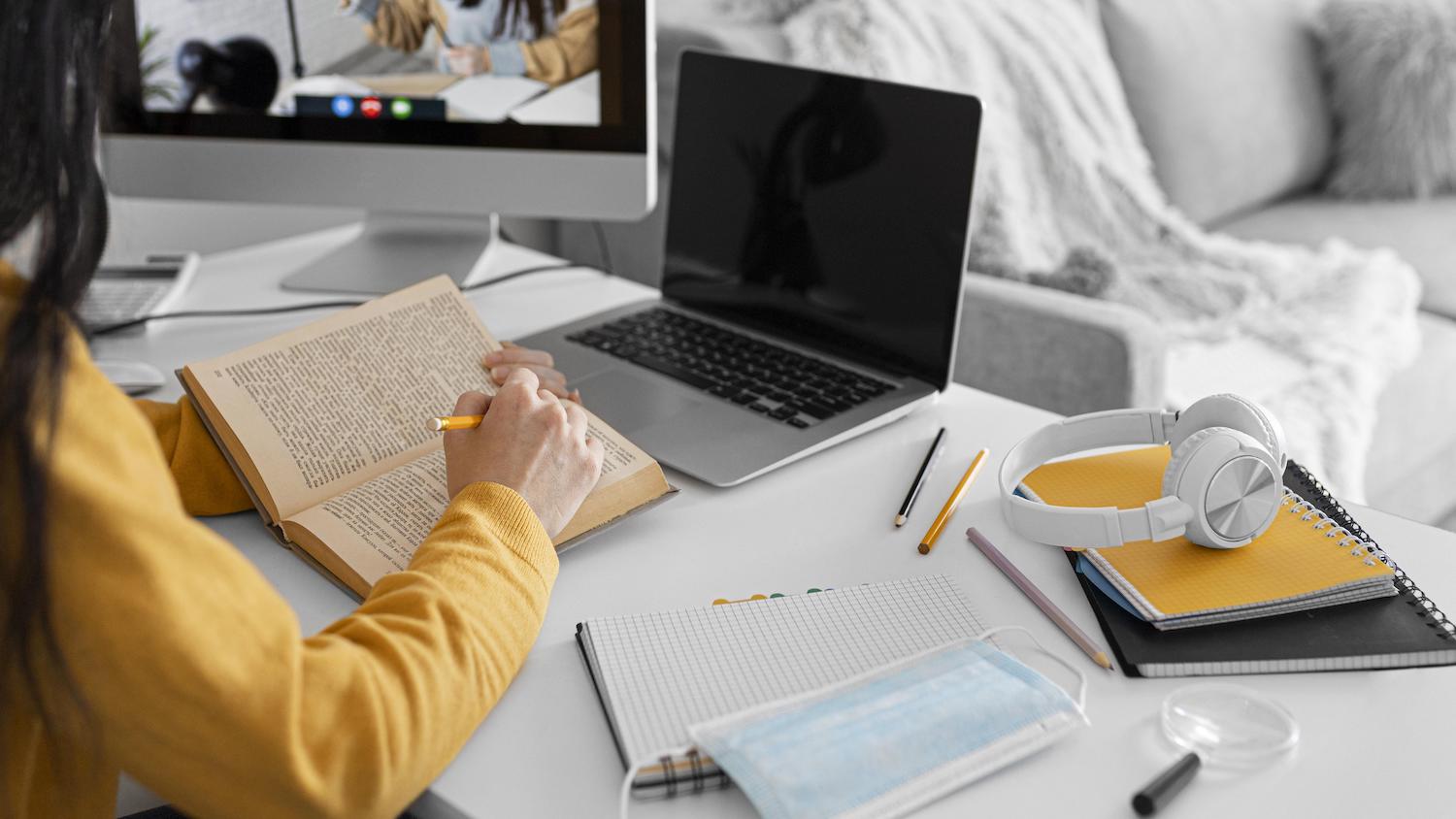
What are you able to do to test the App
- To try the application locally on your computer you may utilize the
PHP expert Serve
command.
This command makes your application browser accessible at http://localhost:8000
. You can test the API endpoints you have developed rate-limiting for via this page by repeatedly calling the purpose of rate-limiting.
The server shows it as the access forbidden result because you've not provided the necessary configuration details on the best option to host your application. Make sure to add these information today.
- Make the
.htaccess
file in the root directory of the app and then add the following code into the file:
RewriteEngine is based on RewriteRule(. *)$ public/$1 [L]
- Make these changes accessible to all users on GitHub and also auto-deployment to carry out the adjustments.
- Open the application via the link provided, and be sure to go to the Laravel Welcome page.
Now it is possible to examine the API endpoints you have implemented rate-limiting with Postman with a series of requests, until you've hit your set limit. It will return an error message that is 4029: To many requests response after exceeding the limit.
Summary
Utilizing features that limit the amount of usage in this Laravel API allows you to regulate the way people use the resources of the application. This will ensure that your users have a secure and reliable experience without spending too much or under. Also, it helps to ensure that your foundational infrastructure is effective and functional.
Marcia Ramos
I'm the editor's team lead at . I'm an advocate of open-source software and love programming. More than seven decades of writing technical documents and editing expertise in the field of technology. I enjoy collaboration with colleagues to create straightforward, easy-to-read documents and improving the efficiency of workflows.
The article was first published here. this site
Article was posted on here